mirror of
https://github.com/puma/puma.git
synced 2022-11-09 13:48:40 -05:00
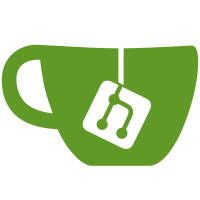
git-svn-id: svn+ssh://rubyforge.org/var/svn/mongrel/trunk@30 19e92222-5c0b-0410-8929-a290d50e31e9
138 lines
3.3 KiB
Text
138 lines
3.3 KiB
Text
require 'rubygems'
|
|
require 'mongrel'
|
|
require 'cgi'
|
|
require 'daemons/daemonize'
|
|
require 'mongrel/command'
|
|
|
|
|
|
class CGIFixed < ::CGI
|
|
public :env_table
|
|
attr_reader :options
|
|
|
|
def initialize(params, data, out, *args)
|
|
@env_table = params
|
|
@args = *args
|
|
@input = StringIO.new(data)
|
|
@out = out
|
|
@options = {}
|
|
super(*args)
|
|
end
|
|
|
|
def header(options = "text/html")
|
|
if options.class == Hash
|
|
# passing in a header so need to keep the status around and other options
|
|
@options = options
|
|
end
|
|
|
|
super(options)
|
|
end
|
|
|
|
def status
|
|
s = @options["Status"] || @options["status"]
|
|
s[0 .. s.index(' ')] || "200"
|
|
end
|
|
|
|
def args
|
|
@args
|
|
end
|
|
|
|
def env_table
|
|
@env_table
|
|
end
|
|
|
|
def stdinput
|
|
@input
|
|
end
|
|
|
|
def stdoutput
|
|
@out
|
|
end
|
|
end
|
|
|
|
|
|
class RailsHandler < Mongrel::HttpHandler
|
|
def initialize(dir)
|
|
@files = Mongrel::DirHandler.new(dir,false)
|
|
@guard = Mutex.new
|
|
end
|
|
|
|
def process(request, response)
|
|
# not static, need to talk to rails
|
|
return if response.socket.closed?
|
|
|
|
if @files.can_serve(request.params["PATH_INFO"])
|
|
@files.process(request,response)
|
|
else
|
|
cgi = CGIFixed.new(request.params, request.body, response.socket)
|
|
begin
|
|
|
|
@guard.synchronize do
|
|
# Rails is not thread safe so must be run entirely within synchronize
|
|
Dispatcher.dispatch(cgi, ActionController::CgiRequest::DEFAULT_SESSION_OPTIONS, response.body)
|
|
end
|
|
|
|
response.status = cgi.status
|
|
response.send_status
|
|
response.send_body
|
|
rescue Object => rails_error
|
|
STDERR.puts "calling Dispatcher.dispatch #{rails_error}"
|
|
STDERR.puts rails_error.backtrace.join("\n")
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
|
|
class StartCommand < Mongrel::Command::Command
|
|
|
|
def configure
|
|
options [
|
|
["-e", "--environment ENV", "Rails environment to run as", :@environment, "production"],
|
|
["-d", "--daemonize", "Whether to run in the background or not", :@daemon, false],
|
|
['-p', '--port PORT', "Which port to bind to", :@port, 3000],
|
|
['-a', '--address ADDR', "Address to bind to", :@address, "0.0.0.0"],
|
|
['-l', '--log FILE', "Where to write log messages", :@log_file, "log/mongrel.log"],
|
|
['-P', '--pid FILE', "Where to write the PID", :@pid_file, "log/mongrel.pid"]
|
|
]
|
|
end
|
|
|
|
def validate
|
|
valid? ["production","development"].include?(@environment), "Only valid environments are 'production' or 'development'"
|
|
valid_dir? File.dirname(@log_file), "Path to log file not valid: #@log_file"
|
|
valid_dir? File.dirname(@pid_file), "Path to pid file not valid: #@pid_file"
|
|
|
|
return @valid
|
|
end
|
|
|
|
|
|
def run
|
|
cwd = Dir.pwd
|
|
|
|
if @daemon
|
|
STDERR.puts "Running as Daemon at #@address:#@port"
|
|
Daemonize.daemonize(log_file=@log_file)
|
|
open(File.join(cwd,@pid_file),"w") {|f| f.write(Process.pid) }
|
|
# change back to the original starting directory
|
|
Dir.chdir(cwd)
|
|
else
|
|
STDERR.puts "Running at #@address:#@port"
|
|
end
|
|
|
|
|
|
require 'config/environment'
|
|
h = Mongrel::HttpServer.new(@address, @port)
|
|
h.register("/", RailsHandler.new(File.join(cwd,"public")))
|
|
h.run
|
|
|
|
begin
|
|
h.acceptor.join
|
|
rescue Interrupt
|
|
STDERR.puts "Interrupted."
|
|
end
|
|
end
|
|
end
|
|
|
|
|
|
|
|
|
|
Mongrel::Command::Registry.instance.run ARGV
|