mirror of
https://github.com/puma/puma.git
synced 2022-11-09 13:48:40 -05:00
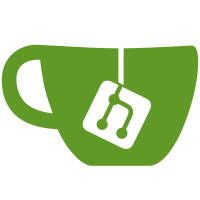
git-svn-id: svn+ssh://rubyforge.org/var/svn/mongrel/trunk@24 19e92222-5c0b-0410-8929-a290d50e31e9
90 lines
1.9 KiB
Text
90 lines
1.9 KiB
Text
require 'rubygems'
|
|
require 'mongrel'
|
|
require 'cgi'
|
|
begin
|
|
require 'daemons/daemonize'
|
|
HAVE_DAEMONS=true
|
|
rescue
|
|
HAVE_DAEMONS=false
|
|
end
|
|
|
|
|
|
class CGIFixed < ::CGI
|
|
public :env_table
|
|
|
|
def initialize(params, data, out, *args)
|
|
@env_table = params
|
|
@args = *args
|
|
@input = StringIO.new(data)
|
|
@out = out
|
|
super(*args)
|
|
end
|
|
|
|
def args
|
|
@args
|
|
end
|
|
|
|
def env_table
|
|
@env_table
|
|
end
|
|
|
|
def stdinput
|
|
@input
|
|
end
|
|
|
|
def stdoutput
|
|
@out
|
|
end
|
|
end
|
|
|
|
|
|
class RailsHandler < Mongrel::HttpHandler
|
|
def initialize(dir)
|
|
@files = Mongrel::DirHandler.new(dir,false)
|
|
@guard = Mutex.new
|
|
end
|
|
|
|
def process(request, response)
|
|
# not static, need to talk to rails
|
|
return if response.socket.closed?
|
|
|
|
if @files.can_serve(request.params["PATH_INFO"])
|
|
@files.process(request,response)
|
|
else
|
|
cgi = CGIFixed.new(request.params, request.body, response.socket)
|
|
begin
|
|
|
|
@guard.synchronize do
|
|
# Rails is not thread safe so must be run entirely within synchronize
|
|
Dispatcher.dispatch(cgi, ActionController::CgiRequest::DEFAULT_SESSION_OPTIONS, response.body)
|
|
end
|
|
|
|
response.send_status
|
|
response.send_body
|
|
rescue IOError
|
|
@log.error("received IOError #$! when handling client. Your web server doesn't like me.")
|
|
rescue Object => rails_error
|
|
@log.error("calling Dispatcher.dispatch", rails_error)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
|
|
if ARGV.length != 2
|
|
STDERR.puts "usage: mongrel_rails <host> <port>"
|
|
exit(1)
|
|
end
|
|
|
|
cwd = Dir.pwd
|
|
|
|
Daemonize.daemonize(log_file=File.join(cwd,"log","mongrel.log"))
|
|
Dir.chdir(cwd) do
|
|
require 'config/environment'
|
|
open(File.join(cwd,"log/mongrel-#{ARGV[1]}.pid"),"w") {|f| f.write(Process.pid) }
|
|
h = Mongrel::HttpServer.new(ARGV[0], ARGV[1])
|
|
h.register("/", RailsHandler.new(File.join(cwd,"public")))
|
|
h.run
|
|
|
|
h.acceptor.join
|
|
end
|