mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
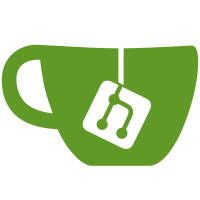
When puma/puma#1403 is merged Puma will support the Early Hints status code for sending assets before a request has finished. While the Early Hints spec is still in draft, this PR prepares Rails to allowing this status code. If the proxy server supports Early Hints, it will send H2 pushes to the client. This PR adds a method for setting Early Hints Link headers via Rails, and also automatically sends Early Hints if supported from the `stylesheet_link_tag` and the `javascript_include_tag`. Once puma supports Early Hints the `--early-hints` argument can be passed to the server to enable this or set in the puma config with `early_hints(true)`. Note that for Early Hints to work in the browser the requirements are 1) a proxy that can handle H2, and 2) HTTPS. To start the server with Early Hints enabled pass `--early-hints` to `rails s`. This has been verified to work with h2o, Puma, and Rails with Chrome. The commit adds a new option to the rails server to enable early hints for Puma. Early Hints spec: https://tools.ietf.org/html/draft-ietf-httpbis-early-hints-04 [Eileen M. Uchitelle, Aaron Patterson]
68 lines
2.8 KiB
Ruby
68 lines
2.8 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "abstract_unit"
|
|
|
|
class JavaScriptHelperTest < ActionView::TestCase
|
|
tests ActionView::Helpers::JavaScriptHelper
|
|
|
|
attr_accessor :output_buffer
|
|
attr_reader :request
|
|
|
|
setup do
|
|
@old_escape_html_entities_in_json = ActiveSupport.escape_html_entities_in_json
|
|
ActiveSupport.escape_html_entities_in_json = true
|
|
@template = self
|
|
@request = Class.new do
|
|
def send_early_hints(links) end
|
|
end.new
|
|
end
|
|
|
|
def teardown
|
|
ActiveSupport.escape_html_entities_in_json = @old_escape_html_entities_in_json
|
|
end
|
|
|
|
def test_escape_javascript
|
|
assert_equal "", escape_javascript(nil)
|
|
assert_equal %(This \\"thing\\" is really\\n netos\\'), escape_javascript(%(This "thing" is really\n netos'))
|
|
assert_equal %(backslash\\\\test), escape_javascript(%(backslash\\test))
|
|
assert_equal %(dont <\\/close> tags), escape_javascript(%(dont </close> tags))
|
|
assert_equal %(unicode 
 newline), escape_javascript(%(unicode \342\200\250 newline).dup.force_encoding(Encoding::UTF_8).encode!)
|
|
assert_equal %(unicode 
 newline), escape_javascript(%(unicode \342\200\251 newline).dup.force_encoding(Encoding::UTF_8).encode!)
|
|
|
|
assert_equal %(dont <\\/close> tags), j(%(dont </close> tags))
|
|
end
|
|
|
|
def test_escape_javascript_with_safebuffer
|
|
given = %('quoted' "double-quoted" new-line:\n </closed>)
|
|
expect = %(\\'quoted\\' \\"double-quoted\\" new-line:\\n <\\/closed>)
|
|
assert_equal expect, escape_javascript(given)
|
|
assert_equal expect, escape_javascript(ActiveSupport::SafeBuffer.new(given))
|
|
assert_instance_of String, escape_javascript(given)
|
|
assert_instance_of ActiveSupport::SafeBuffer, escape_javascript(ActiveSupport::SafeBuffer.new(given))
|
|
end
|
|
|
|
def test_javascript_tag
|
|
self.output_buffer = "foo"
|
|
|
|
assert_dom_equal "<script>\n//<![CDATA[\nalert('hello')\n//]]>\n</script>",
|
|
javascript_tag("alert('hello')")
|
|
|
|
assert_equal "foo", output_buffer, "javascript_tag without a block should not concat to output_buffer"
|
|
end
|
|
|
|
# Setting the :extname option will control what extension (if any) is appended to the url for assets
|
|
def test_javascript_include_tag
|
|
assert_dom_equal "<script src='/foo.js'></script>", javascript_include_tag("/foo")
|
|
assert_dom_equal "<script src='/foo'></script>", javascript_include_tag("/foo", extname: false)
|
|
assert_dom_equal "<script src='/foo.bar'></script>", javascript_include_tag("/foo", extname: ".bar")
|
|
end
|
|
|
|
def test_javascript_tag_with_options
|
|
assert_dom_equal "<script id=\"the_js_tag\">\n//<![CDATA[\nalert('hello')\n//]]>\n</script>",
|
|
javascript_tag("alert('hello')", id: "the_js_tag")
|
|
end
|
|
|
|
def test_javascript_cdata_section
|
|
assert_dom_equal "\n//<![CDATA[\nalert('hello')\n//]]>\n", javascript_cdata_section("alert('hello')")
|
|
end
|
|
end
|