mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
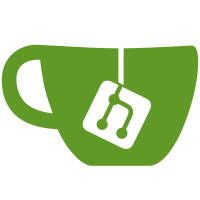
We shouldn't depend on specific methods imlemented in the TestResponse subclass because the response could actually be a real response object. In the future, we should either push the aliased predicate methods in TestResponse up to the real response object, or remove them
63 lines
1.6 KiB
Ruby
63 lines
1.6 KiB
Ruby
require 'abstract_unit'
|
|
require 'action_dispatch/testing/assertions/response'
|
|
|
|
module ActionDispatch
|
|
module Assertions
|
|
class ResponseAssertionsTest < ActiveSupport::TestCase
|
|
include ResponseAssertions
|
|
|
|
FakeResponse = Struct.new(:response_code) do
|
|
[:successful, :not_found, :redirection, :server_error].each do |sym|
|
|
define_method("#{sym}?") do
|
|
sym == response_code
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_assert_response_predicate_methods
|
|
[:success, :missing, :redirect, :error].each do |sym|
|
|
@response = FakeResponse.new RESPONSE_PREDICATES[sym].to_s.sub(/\?/, '').to_sym
|
|
assert_response sym
|
|
|
|
assert_raises(Minitest::Assertion) {
|
|
assert_response :unauthorized
|
|
}
|
|
end
|
|
end
|
|
|
|
def test_assert_response_fixnum
|
|
@response = FakeResponse.new 400
|
|
assert_response 400
|
|
|
|
assert_raises(Minitest::Assertion) {
|
|
assert_response :unauthorized
|
|
}
|
|
|
|
assert_raises(Minitest::Assertion) {
|
|
assert_response 500
|
|
}
|
|
end
|
|
|
|
def test_assert_response_sym_status
|
|
@response = FakeResponse.new 401
|
|
assert_response :unauthorized
|
|
|
|
assert_raises(Minitest::Assertion) {
|
|
assert_response :ok
|
|
}
|
|
|
|
assert_raises(Minitest::Assertion) {
|
|
assert_response :success
|
|
}
|
|
end
|
|
|
|
def test_assert_response_sym_typo
|
|
@response = FakeResponse.new 200
|
|
|
|
assert_raises(ArgumentError) {
|
|
assert_response :succezz
|
|
}
|
|
end
|
|
end
|
|
end
|
|
end
|