mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
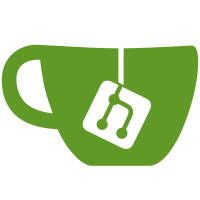
In Rails 4.1, Action View was extracted from Action Pack, but this change was not reflected in the API documentation of ActiveModel::Model. This commits makes it explicit in the documentation that Active Model also interacts with Action View as well as Action Pack. [ci skip]
97 lines
2.7 KiB
Ruby
97 lines
2.7 KiB
Ruby
module ActiveModel
|
|
# == Active \Model \Basic \Model
|
|
#
|
|
# Includes the required interface for an object to interact with
|
|
# Action Pack and Action View, using different Active Model modules.
|
|
# It includes model name introspections, conversions, translations and
|
|
# validations. Besides that, it allows you to initialize the object with a
|
|
# hash of attributes, pretty much like Active Record does.
|
|
#
|
|
# A minimal implementation could be:
|
|
#
|
|
# class Person
|
|
# include ActiveModel::Model
|
|
# attr_accessor :name, :age
|
|
# end
|
|
#
|
|
# person = Person.new(name: 'bob', age: '18')
|
|
# person.name # => "bob"
|
|
# person.age # => "18"
|
|
#
|
|
# Note that, by default, <tt>ActiveModel::Model</tt> implements <tt>persisted?</tt>
|
|
# to return +false+, which is the most common case. You may want to override
|
|
# it in your class to simulate a different scenario:
|
|
#
|
|
# class Person
|
|
# include ActiveModel::Model
|
|
# attr_accessor :id, :name
|
|
#
|
|
# def persisted?
|
|
# self.id == 1
|
|
# end
|
|
# end
|
|
#
|
|
# person = Person.new(id: 1, name: 'bob')
|
|
# person.persisted? # => true
|
|
#
|
|
# Also, if for some reason you need to run code on <tt>initialize</tt>, make
|
|
# sure you call +super+ if you want the attributes hash initialization to
|
|
# happen.
|
|
#
|
|
# class Person
|
|
# include ActiveModel::Model
|
|
# attr_accessor :id, :name, :omg
|
|
#
|
|
# def initialize(attributes={})
|
|
# super
|
|
# @omg ||= true
|
|
# end
|
|
# end
|
|
#
|
|
# person = Person.new(id: 1, name: 'bob')
|
|
# person.omg # => true
|
|
#
|
|
# For more detailed information on other functionalities available, please
|
|
# refer to the specific modules included in <tt>ActiveModel::Model</tt>
|
|
# (see below).
|
|
module Model
|
|
extend ActiveSupport::Concern
|
|
include ActiveModel::AttributeAssignment
|
|
include ActiveModel::Validations
|
|
include ActiveModel::Conversion
|
|
|
|
included do
|
|
extend ActiveModel::Naming
|
|
extend ActiveModel::Translation
|
|
end
|
|
|
|
# Initializes a new model with the given +params+.
|
|
#
|
|
# class Person
|
|
# include ActiveModel::Model
|
|
# attr_accessor :name, :age
|
|
# end
|
|
#
|
|
# person = Person.new(name: 'bob', age: '18')
|
|
# person.name # => "bob"
|
|
# person.age # => "18"
|
|
def initialize(attributes = {})
|
|
assign_attributes(attributes) if attributes
|
|
|
|
super()
|
|
end
|
|
|
|
# Indicates if the model is persisted. Default is +false+.
|
|
#
|
|
# class Person
|
|
# include ActiveModel::Model
|
|
# attr_accessor :id, :name
|
|
# end
|
|
#
|
|
# person = Person.new(id: 1, name: 'bob')
|
|
# person.persisted? # => false
|
|
def persisted?
|
|
false
|
|
end
|
|
end
|
|
end
|