mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
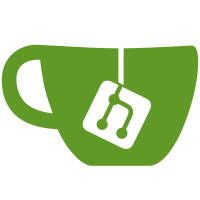
* Add performance benchmark similar to ActiveRecord * Lazily find_or_create_resource_for_collection to not incur the overhead for empty arrays and arrays of primatives * #duplicable? is faster than inline rescues when the object is not duplicable * Don't constantly raise and handle NameError, raising is expensive * Even when a resource is nested inside a module, always look inside the class first for the resource definition so we don't overwrite classes all the time Before: user system total real Model.new (instantiation) 0.120000 0.000000 0.120000 ( 0.119961) Nested::Model.new (instantiation) 0.150000 0.010000 0.160000 ( 0.151183) Model.new (setting attributes) 28.540000 0.680000 29.220000 ( 29.271775) Nested::Model.new (setting attributes) 29.740000 0.580000 30.320000 ( 30.486210) After: user system total real Model.new (instantiation) 0.120000 0.000000 0.120000 ( 0.121249) Nested::Model.new (instantiation) 0.150000 0.010000 0.160000 ( 0.152429) Model.new (setting attributes) 11.480000 0.170000 11.650000 ( 11.656163) Nested::Model.new (setting attributes) 11.510000 0.210000 11.720000 ( 11.724249)
70 lines
1.7 KiB
Ruby
70 lines
1.7 KiB
Ruby
require 'rubygems'
|
|
require 'active_resource'
|
|
require 'benchmark'
|
|
|
|
TIMES = (ENV['N'] || 10_000).to_i
|
|
|
|
# deep nested resource
|
|
attrs = {
|
|
:id => 1,
|
|
:name => 'Luis',
|
|
:age => 21,
|
|
:friends => [
|
|
{
|
|
:name => 'JK',
|
|
:age => 24,
|
|
:colors => ['red', 'green', 'blue'],
|
|
:brothers => [
|
|
{
|
|
:name => 'Mateo',
|
|
:age => 35,
|
|
:children => [{ :name => 'Edith', :age => 5 }, { :name => 'Martha', :age => 4 }]
|
|
},
|
|
{
|
|
:name => 'Felipe',
|
|
:age => 33,
|
|
:children => [{ :name => 'Bryan', :age => 1 }, { :name => 'Luke', :age => 0 }]
|
|
}
|
|
]
|
|
},
|
|
{
|
|
:name => 'Eduardo',
|
|
:age => 20,
|
|
:colors => [],
|
|
:brothers => [
|
|
{
|
|
:name => 'Sebas',
|
|
:age => 23,
|
|
:children => [{ :name => 'Andres', :age => 0 }, { :name => 'Jorge', :age => 2 }]
|
|
},
|
|
{
|
|
:name => 'Elsa',
|
|
:age => 19,
|
|
:children => [{ :name => 'Natacha', :age => 1 }]
|
|
},
|
|
{
|
|
:name => 'Milena',
|
|
:age => 16,
|
|
:children => []
|
|
}
|
|
]
|
|
}
|
|
]
|
|
}
|
|
|
|
class Customer < ActiveResource::Base
|
|
self.site = "http://37s.sunrise.i:3000"
|
|
end
|
|
|
|
module Nested
|
|
class Customer < ActiveResource::Base
|
|
self.site = "http://37s.sunrise.i:3000"
|
|
end
|
|
end
|
|
|
|
Benchmark.bm(40) do |x|
|
|
x.report('Model.new (instantiation)') { TIMES.times { Customer.new } }
|
|
x.report('Nested::Model.new (instantiation)') { TIMES.times { Nested::Customer.new } }
|
|
x.report('Model.new (setting attributes)') { TIMES.times { Customer.new attrs } }
|
|
x.report('Nested::Model.new (setting attributes)') { TIMES.times { Nested::Customer.new attrs } }
|
|
end
|