mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
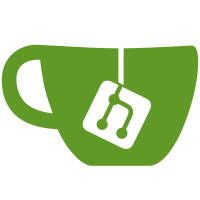
Instead of treating it as an anonymous block, execute the `ActionView::Base.field_error_proc` within the context of the `ActionView::Base` instance. This enables consumer applications to continue to override the proc as they see fit, but frees them from declaring templating logic within a `config/initializers/*.rb`, `config/environments/*.rb` or `config/application.rb` file. This makes it possible to replace something like: ```ruby config.action_view.field_error_proc = proc do |html_tag, instance| <<~HTML.html_safe #{html_tag} <span class="errors">#{instance.error_message.to_sentence}</span> HTML end ``` With inline calls to Action View helpers like: ```ruby config.action_view.field_error_proc = proc do |html_tag, instance| safe_join [ html_tag, tag.span(instance.error_message.to_sentence, class: "errors") ] end ``` Or with a view partial rendering, like: ```ruby config.action_view.field_error_proc = proc do |html_tag, instance| render partial: "application/field_with_errors", locals: { html_tag: html_tag, instance: instance } end ``` Then, elsewhere in `app/views/application/field_with_errors.html.erb`: ```erb <%= html_tag %> <span class="errors"><%= instance.error_message.to_sentence %></span> ```
54 lines
1.3 KiB
Ruby
54 lines
1.3 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "active_support/core_ext/module/attribute_accessors"
|
|
require "active_support/core_ext/enumerable"
|
|
|
|
module ActionView
|
|
# = Active Model Helpers
|
|
module Helpers # :nodoc:
|
|
module ActiveModelHelper
|
|
end
|
|
|
|
module ActiveModelInstanceTag
|
|
def object
|
|
@active_model_object ||= begin
|
|
object = super
|
|
object.respond_to?(:to_model) ? object.to_model : object
|
|
end
|
|
end
|
|
|
|
def content_tag(type, options, *)
|
|
select_markup_helper?(type) ? super : error_wrapping(super)
|
|
end
|
|
|
|
def tag(type, options, *)
|
|
tag_generate_errors?(options) ? error_wrapping(super) : super
|
|
end
|
|
|
|
def error_wrapping(html_tag)
|
|
if object_has_errors?
|
|
@template_object.instance_exec(html_tag, self, &Base.field_error_proc)
|
|
else
|
|
html_tag
|
|
end
|
|
end
|
|
|
|
def error_message
|
|
object.errors[@method_name]
|
|
end
|
|
|
|
private
|
|
def object_has_errors?
|
|
object.respond_to?(:errors) && object.errors.respond_to?(:[]) && error_message.present?
|
|
end
|
|
|
|
def select_markup_helper?(type)
|
|
["optgroup", "option"].include?(type)
|
|
end
|
|
|
|
def tag_generate_errors?(options)
|
|
options["type"] != "hidden"
|
|
end
|
|
end
|
|
end
|
|
end
|