mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
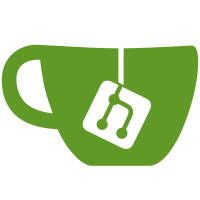
* before_configuration: this hook is run immediately after the Application class comes into existence, but before the user has added any configuration. This is the appropriate place to set configuration for your plugin * before_initialize: This is run after all of the user's configuration has completed, but before any initializers have begun (in other words, it runs right after config/environments/{development,production,test}.rb) * after_initialize: This is run after all of the initializers have run. It is an appropriate place for forking in a preforking setup Each of these hooks may be used via ActiveSupport.on_load(name) { }. In all these cases, the context inside the block will be the Application object. This means that for simple cases, you can use these hooks without needing to create a Railtie.
74 lines
No EOL
2.7 KiB
Ruby
74 lines
No EOL
2.7 KiB
Ruby
require "active_support/notifications"
|
|
|
|
module Rails
|
|
class Application
|
|
module Bootstrap
|
|
include Initializable
|
|
|
|
initializer :load_environment_config do
|
|
environment = config.paths.config.environments.to_a.first
|
|
require environment if environment
|
|
end
|
|
|
|
initializer :load_active_support do
|
|
require 'active_support/dependencies'
|
|
require "active_support/all" unless config.active_support.bare
|
|
end
|
|
|
|
# Preload all frameworks specified by the Configuration#frameworks.
|
|
# Used by Passenger to ensure everything's loaded before forking and
|
|
# to avoid autoload race conditions in JRuby.
|
|
initializer :preload_frameworks do
|
|
ActiveSupport::Autoload.eager_autoload! if config.preload_frameworks
|
|
end
|
|
|
|
# Initialize the logger early in the stack in case we need to log some deprecation.
|
|
initializer :initialize_logger do
|
|
Rails.logger ||= config.logger || begin
|
|
path = config.paths.log.to_a.first
|
|
logger = ActiveSupport::BufferedLogger.new(path)
|
|
logger.level = ActiveSupport::BufferedLogger.const_get(config.log_level.to_s.upcase)
|
|
logger.auto_flushing = false if Rails.env.production?
|
|
logger
|
|
rescue StandardError => e
|
|
logger = ActiveSupport::BufferedLogger.new(STDERR)
|
|
logger.level = ActiveSupport::BufferedLogger::WARN
|
|
logger.warn(
|
|
"Rails Error: Unable to access log file. Please ensure that #{path} exists and is chmod 0666. " +
|
|
"The log level has been raised to WARN and the output directed to STDERR until the problem is fixed."
|
|
)
|
|
logger
|
|
end
|
|
end
|
|
|
|
# Initialize cache early in the stack so railties can make use of it.
|
|
initializer :initialize_cache do
|
|
unless defined?(RAILS_CACHE)
|
|
silence_warnings { Object.const_set "RAILS_CACHE", ActiveSupport::Cache.lookup_store(config.cache_store) }
|
|
|
|
if RAILS_CACHE.respond_to?(:middleware)
|
|
config.middleware.insert_after(:"Rack::Lock", RAILS_CACHE.middleware)
|
|
end
|
|
end
|
|
end
|
|
|
|
initializer :set_clear_dependencies_hook do
|
|
unless config.cache_classes
|
|
ActionDispatch::Callbacks.after do
|
|
ActiveSupport::Dependencies.clear
|
|
end
|
|
end
|
|
end
|
|
|
|
# Sets the dependency loading mechanism.
|
|
# TODO: Remove files from the $" and always use require.
|
|
initializer :initialize_dependency_mechanism do
|
|
ActiveSupport::Dependencies.mechanism = config.cache_classes ? :require : :load
|
|
end
|
|
|
|
initializer :bootstrap_hook do |app|
|
|
ActiveSupport.run_load_hooks(:before_initialize, app)
|
|
end
|
|
end
|
|
end
|
|
end |