mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
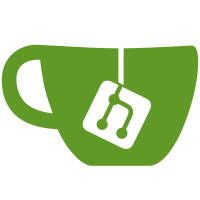
`Dir.glob` doesn't guarantee the order of its results: https://ruby-doc.org/core-2.6.5/Dir.html#method-c-glob > Case sensitivity depends on your system (File::FNM_CASEFOLD is > ignored), as does the order in which the results are returned. Minitest stores a list of all test cases in the order that they were defined; it shuffles them before they're run, but doesn't sort them: https://github.com/seattlerb/minitest/blob/v5.13.0/lib/minitest.rb#L1048 https://github.com/seattlerb/minitest/blob/v5.13.0/lib/minitest.rb#L156 This means that the order in which framework tests run is platform dependent, and running a test command that failed in CI locally won't necessarily reproduce the error, even when the same seed is provided. `Rake::FileList` resolves glob patterns to a sorted list of files: https://github.com/ruby/rake/blob/v13.0.1/lib/rake/file_list.rb#L408 By using `Rake::FileList` instead of `Dir.glob`, framework tests will always run in the same order when given the same seed, and reproducing order dependent CI failures will be easier.
158 lines
3.8 KiB
Ruby
158 lines
3.8 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "rake/testtask"
|
|
require "fileutils"
|
|
require "open3"
|
|
|
|
desc "Default Task"
|
|
task default: :test
|
|
|
|
task package: %w( assets:compile assets:verify )
|
|
|
|
# Run the unit tests
|
|
|
|
desc "Run all unit tests"
|
|
task test: ["test:template", "test:integration:action_pack", "test:integration:active_record"]
|
|
|
|
namespace :test do
|
|
task :isolated do
|
|
Dir.glob("test/{actionpack,activerecord,template}/**/*_test.rb").all? do |file|
|
|
sh(Gem.ruby, "-w", "-Ilib:test", file)
|
|
end || raise("Failures")
|
|
end
|
|
|
|
Rake::TestTask.new(:template) do |t|
|
|
t.libs << "test"
|
|
t.test_files = FileList["test/template/**/*_test.rb"]
|
|
t.warning = true
|
|
t.verbose = true
|
|
t.ruby_opts = ["--dev"] if defined?(JRUBY_VERSION)
|
|
end
|
|
|
|
desc "Run tests for rails-ujs"
|
|
task :ujs do
|
|
system("npm run lint")
|
|
exit $?.exitstatus unless $?.success?
|
|
|
|
begin
|
|
listen_host = "localhost"
|
|
listen_port = "4567"
|
|
|
|
runner_command = %w(ruby ../ci/qunit-selenium-runner.rb)
|
|
if ENV["SELENIUM_DRIVER_URL"]
|
|
require "socket"
|
|
runner_command += %W(http://#{Socket.gethostname}:#{listen_port}/ #{ENV["SELENIUM_DRIVER_URL"]})
|
|
listen_host = "0.0.0.0"
|
|
else
|
|
runner_command += %W(http://localhost:#{listen_port}/)
|
|
end
|
|
|
|
Dir.mkdir("log")
|
|
pid = File.open("log/test.log", "w") do |f|
|
|
spawn(*%W(rackup test/ujs/config.ru -o #{listen_host} -p #{listen_port} -s puma), out: f, err: f, pgroup: true)
|
|
end
|
|
|
|
start_time = Time.now
|
|
|
|
loop do
|
|
break if system("lsof -i :4567", 1 => File::NULL)
|
|
|
|
if Time.now - start_time > 5
|
|
puts "Failed to start puma after 5 seconds"
|
|
puts
|
|
puts File.read("log/test.log")
|
|
exit 1
|
|
end
|
|
|
|
sleep 0.2
|
|
end
|
|
|
|
system(*runner_command)
|
|
status = $?.exitstatus
|
|
ensure
|
|
Process.kill("KILL", -pid) if pid
|
|
FileUtils.rm_rf("log")
|
|
end
|
|
|
|
exit status
|
|
end
|
|
|
|
namespace :integration do
|
|
# Active Record Integration Tests
|
|
Rake::TestTask.new(:active_record) do |t|
|
|
t.libs << "test"
|
|
t.test_files = FileList["test/activerecord/*_test.rb"]
|
|
t.warning = true
|
|
t.verbose = true
|
|
t.ruby_opts = ["--dev"] if defined?(JRUBY_VERSION)
|
|
end
|
|
|
|
# Action Pack Integration Tests
|
|
Rake::TestTask.new(:action_pack) do |t|
|
|
t.libs << "test"
|
|
t.test_files = FileList["test/actionpack/**/*_test.rb"]
|
|
t.warning = true
|
|
t.verbose = true
|
|
t.ruby_opts = ["--dev"] if defined?(JRUBY_VERSION)
|
|
end
|
|
end
|
|
end
|
|
|
|
namespace :ujs do
|
|
desc "Starts the test server"
|
|
task :server do
|
|
system "bundle exec rackup test/ujs/config.ru -p 4567 -s puma"
|
|
end
|
|
end
|
|
|
|
namespace :assets do
|
|
desc "Compile Action View assets"
|
|
task :compile do
|
|
require "blade"
|
|
require "sprockets"
|
|
require "sprockets/export"
|
|
Blade.build
|
|
end
|
|
|
|
desc "Verify compiled Action View assets"
|
|
task :verify do
|
|
file = "lib/assets/compiled/rails-ujs.js"
|
|
pathname = Pathname.new("#{__dir__}/#{file}")
|
|
|
|
print "[verify] #{file} exists "
|
|
if pathname.exist?
|
|
puts "[OK]"
|
|
else
|
|
$stderr.puts "[FAIL]"
|
|
fail
|
|
end
|
|
|
|
print "[verify] #{file} is a UMD module "
|
|
if /module\.exports.*define\.amd/m.match?(pathname.read)
|
|
puts "[OK]"
|
|
else
|
|
$stderr.puts "[FAIL]"
|
|
fail
|
|
end
|
|
|
|
print "[verify] #{__dir__} can be required as a module "
|
|
js = <<-JS
|
|
window = { Event: class {} }
|
|
class Element {}
|
|
require('#{__dir__}')
|
|
JS
|
|
_, stderr, status = Open3.capture3("node", "--print", js)
|
|
if status.success?
|
|
puts "[OK]"
|
|
else
|
|
$stderr.puts "[FAIL]\n#{stderr}"
|
|
fail
|
|
end
|
|
end
|
|
end
|
|
|
|
task :lines do
|
|
load File.expand_path("../tools/line_statistics", __dir__)
|
|
files = FileList["lib/**/*.rb"]
|
|
CodeTools::LineStatistics.new(files).print_loc
|
|
end
|