mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
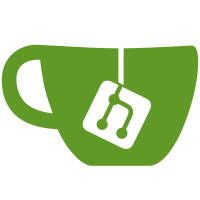
The Logger by default includes a guard which checks for the logging level. By removing the custom logging guards, we can decouple the logging guard from the logging action to be done. This also follows the good practice listed on http://guides.rubyonrails.org/debugging_rails_applications.html#impact-of-logs-on-performance.
39 lines
1 KiB
Ruby
39 lines
1 KiB
Ruby
require 'active_support/log_subscriber'
|
|
|
|
module ActionMailer
|
|
# Implements the ActiveSupport::LogSubscriber for logging notifications when
|
|
# email is delivered and received.
|
|
class LogSubscriber < ActiveSupport::LogSubscriber
|
|
# An email was delivered.
|
|
def deliver(event)
|
|
info do
|
|
recipients = Array(event.payload[:to]).join(', ')
|
|
"\nSent mail to #{recipients} (#{event.duration.round(1)}ms)"
|
|
end
|
|
|
|
debug { event.payload[:mail] }
|
|
end
|
|
|
|
# An email was received.
|
|
def receive(event)
|
|
info { "\nReceived mail (#{event.duration.round(1)}ms)" }
|
|
debug { event.payload[:mail] }
|
|
end
|
|
|
|
# An email was generated.
|
|
def process(event)
|
|
debug do
|
|
mailer = event.payload[:mailer]
|
|
action = event.payload[:action]
|
|
"\n#{mailer}##{action}: processed outbound mail in #{event.duration.round(1)}ms"
|
|
end
|
|
end
|
|
|
|
# Use the logger configured for ActionMailer::Base
|
|
def logger
|
|
ActionMailer::Base.logger
|
|
end
|
|
end
|
|
end
|
|
|
|
ActionMailer::LogSubscriber.attach_to :action_mailer
|