mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
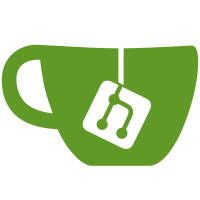
* Specify accept-charset on all forms. All recent browsers, as well as IE5+, will use the encoding specified for form parameters * Unfortunately, IE5+ will not look at accept-charset unless at least one character in the form's values is not in the page's charset. Since the user can override the default charset (which Rails sets to UTF-8), we provide a hidden input containing a unicode character, forcing IE to look at the accept-charset. * Now that the vast majority of web input is UTF-8, we set the inbound parameters to UTF-8. This will eliminate many cases of incompatible encodings between ASCII-8BIT and UTF-8. * You can safely ignore params[:_snowman_] TODO: * Validate inbound text to confirm it is UTF-8 * Combine the whole_form implementations in form_helper_test and form_tag_helper_test
79 lines
2.4 KiB
Ruby
79 lines
2.4 KiB
Ruby
require 'active_support/core_ext/hash/keys'
|
|
require 'active_support/core_ext/hash/indifferent_access'
|
|
|
|
module ActionDispatch
|
|
module Http
|
|
module Parameters
|
|
# Returns both GET and POST \parameters in a single hash.
|
|
def parameters
|
|
@env["action_dispatch.request.parameters"] ||= begin
|
|
params = request_parameters.merge(query_parameters)
|
|
params.merge!(path_parameters)
|
|
encode_params(params).with_indifferent_access
|
|
end
|
|
end
|
|
alias :params :parameters
|
|
|
|
def path_parameters=(parameters) #:nodoc:
|
|
@env.delete("action_dispatch.request.symbolized_path_parameters")
|
|
@env.delete("action_dispatch.request.parameters")
|
|
@env["action_dispatch.request.path_parameters"] = parameters
|
|
end
|
|
|
|
# The same as <tt>path_parameters</tt> with explicitly symbolized keys.
|
|
def symbolized_path_parameters
|
|
@env["action_dispatch.request.symbolized_path_parameters"] ||= path_parameters.symbolize_keys
|
|
end
|
|
|
|
# Returns a hash with the \parameters used to form the \path of the request.
|
|
# Returned hash keys are strings:
|
|
#
|
|
# {'action' => 'my_action', 'controller' => 'my_controller'}
|
|
#
|
|
# See <tt>symbolized_path_parameters</tt> for symbolized keys.
|
|
def path_parameters
|
|
@env["action_dispatch.request.path_parameters"] ||= {}
|
|
end
|
|
|
|
private
|
|
|
|
# TODO: Validate that the characters are UTF-8. If they aren't,
|
|
# you'll get a weird error down the road, but our form handling
|
|
# should really prevent that from happening
|
|
def encode_params(params)
|
|
return params unless "ruby".encoding_aware?
|
|
|
|
if params.is_a?(String)
|
|
return params.force_encoding("UTF-8").encode!
|
|
elsif !params.is_a?(Hash)
|
|
return params
|
|
end
|
|
|
|
params.each do |k, v|
|
|
case v
|
|
when Hash
|
|
encode_params(v)
|
|
when Array
|
|
v.map! {|el| encode_params(el) }
|
|
else
|
|
encode_params(v)
|
|
end
|
|
end
|
|
end
|
|
|
|
# Convert nested Hash to HashWithIndifferentAccess
|
|
def normalize_parameters(value)
|
|
case value
|
|
when Hash
|
|
h = {}
|
|
value.each { |k, v| h[k] = normalize_parameters(v) }
|
|
h.with_indifferent_access
|
|
when Array
|
|
value.map { |e| normalize_parameters(e) }
|
|
else
|
|
value
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|