mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
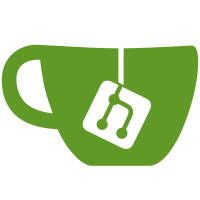
Previously we would just silently write the attribute. This can lead to subtle bugs (for example, see the change in AutosaveAssociation where a through association would wrongly gain an attribute. Also, ensuring that we never gain any new attributes after initialization will allow me to reduce our dependence on method_missing.
25 lines
691 B
Ruby
25 lines
691 B
Ruby
class Contact < ActiveRecord::Base
|
|
establish_connection(:adapter => 'fake')
|
|
|
|
connection.tables = ['contacts']
|
|
connection.primary_keys = {
|
|
'contacts' => 'id'
|
|
}
|
|
|
|
# mock out self.columns so no pesky db is needed for these tests
|
|
def self.column(name, sql_type = nil, options = {})
|
|
connection.merge_column('contacts', name, sql_type, options)
|
|
end
|
|
|
|
column :name, :string
|
|
column :age, :integer
|
|
column :avatar, :binary
|
|
column :created_at, :datetime
|
|
column :awesome, :boolean
|
|
column :preferences, :string
|
|
column :alternative_id, :integer
|
|
|
|
serialize :preferences
|
|
|
|
belongs_to :alternative, :class_name => 'Contact'
|
|
end
|