mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
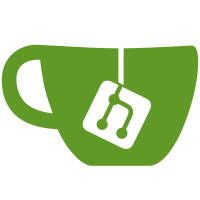
Applying `includes` and `joins` to a relation that selected additional database fields would cause those additional fields not to be included in the results even though they were queried from the database: posts = Post.select('1 as other').includes(:tbl).joins(:tbl) posts.to_sql.include?('1 as other') #=> true posts.first.attributes.include?('other') #=> false This commit includes these additionally selected fields in the instantiated results.
43 lines
1.3 KiB
Ruby
43 lines
1.3 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "cases/helper"
|
|
require "models/post"
|
|
require "models/comment"
|
|
|
|
module ActiveRecord
|
|
class SelectTest < ActiveRecord::TestCase
|
|
fixtures :posts, :comments
|
|
|
|
def test_select_with_nil_argument
|
|
expected = Post.select(:title).to_sql
|
|
assert_equal expected, Post.select(nil).select(:title).to_sql
|
|
end
|
|
|
|
def test_reselect
|
|
expected = Post.select(:title).to_sql
|
|
assert_equal expected, Post.select(:title, :body).reselect(:title).to_sql
|
|
end
|
|
|
|
def test_reselect_with_default_scope_select
|
|
expected = Post.select(:title).to_sql
|
|
actual = PostWithDefaultSelect.reselect(:title).to_sql
|
|
|
|
assert_equal expected, actual
|
|
end
|
|
|
|
def test_aliased_select_using_as_with_joins_and_includes
|
|
posts = Post.select("posts.id AS field_alias").joins(:comments).includes(:comments)
|
|
assert_includes posts.first.attributes, "field_alias"
|
|
end
|
|
|
|
def test_aliased_select_not_using_as_with_joins_and_includes
|
|
posts = Post.select("posts.id field_alias").joins(:comments).includes(:comments)
|
|
assert_includes posts.first.attributes, "field_alias"
|
|
end
|
|
|
|
def test_star_select_with_joins_and_includes
|
|
posts = Post.select("posts.*").joins(:comments).includes(:comments)
|
|
assert_not_includes posts.first.attributes, "posts.*"
|
|
end
|
|
end
|
|
end
|