mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
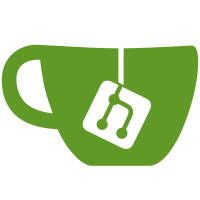
The log output used to be confusing in situation where type casting has "unexpected" effects. For example when finding records with a `String`. BEFORE: irb(main):002:0> Event.find("im-no-integer") D, [2013-11-09T11:10:28.998857 #1706] DEBUG -- : Event Load (4.5ms) SELECT "events".* FROM "events" WHERE "events"."id" = $1 LIMIT 1 [["id", "im-no-integer"]] AFTER: irb(main):002:0> Event.find("im-no-integer") D, [2013-11-09T11:10:28.998857 #1706] DEBUG -- : Event Load (4.5ms) SELECT "events".* FROM "events" WHERE "events"."id" = $1 LIMIT 1 [["id", 0]]
94 lines
2.3 KiB
Ruby
94 lines
2.3 KiB
Ruby
require 'cases/helper'
|
|
require 'models/topic'
|
|
|
|
module ActiveRecord
|
|
class BindParameterTest < ActiveRecord::TestCase
|
|
fixtures :topics
|
|
|
|
class LogListener
|
|
attr_accessor :calls
|
|
|
|
def initialize
|
|
@calls = []
|
|
end
|
|
|
|
def call(*args)
|
|
calls << args
|
|
end
|
|
end
|
|
|
|
def setup
|
|
super
|
|
@connection = ActiveRecord::Base.connection
|
|
@listener = LogListener.new
|
|
@pk = Topic.columns.find { |c| c.primary }
|
|
ActiveSupport::Notifications.subscribe('sql.active_record', @listener)
|
|
end
|
|
|
|
def teardown
|
|
ActiveSupport::Notifications.unsubscribe(@listener)
|
|
end
|
|
|
|
if ActiveRecord::Base.connection.supports_statement_cache?
|
|
def test_binds_are_logged
|
|
sub = @connection.substitute_at(@pk, 0)
|
|
binds = [[@pk, 1]]
|
|
sql = "select * from topics where id = #{sub}"
|
|
|
|
@connection.exec_query(sql, 'SQL', binds)
|
|
|
|
message = @listener.calls.find { |args| args[4][:sql] == sql }
|
|
assert_equal binds, message[4][:binds]
|
|
end
|
|
|
|
def test_binds_are_logged_after_type_cast
|
|
sub = @connection.substitute_at(@pk, 0)
|
|
binds = [[@pk, "3"]]
|
|
sql = "select * from topics where id = #{sub}"
|
|
|
|
@connection.exec_query(sql, 'SQL', binds)
|
|
|
|
message = @listener.calls.find { |args| args[4][:sql] == sql }
|
|
assert_equal [[@pk, 3]], message[4][:binds]
|
|
end
|
|
|
|
def test_find_one_uses_binds
|
|
Topic.find(1)
|
|
binds = [[@pk, 1]]
|
|
message = @listener.calls.find { |args| args[4][:binds] == binds }
|
|
assert message, 'expected a message with binds'
|
|
end
|
|
|
|
def test_logs_bind_vars
|
|
pk = Topic.columns.find { |x| x.primary }
|
|
|
|
payload = {
|
|
:name => 'SQL',
|
|
:sql => 'select * from topics where id = ?',
|
|
:binds => [[pk, 10]]
|
|
}
|
|
event = ActiveSupport::Notifications::Event.new(
|
|
'foo',
|
|
Time.now,
|
|
Time.now,
|
|
123,
|
|
payload)
|
|
|
|
logger = Class.new(ActiveRecord::LogSubscriber) {
|
|
attr_reader :debugs
|
|
def initialize
|
|
super
|
|
@debugs = []
|
|
end
|
|
|
|
def debug str
|
|
@debugs << str
|
|
end
|
|
}.new
|
|
|
|
logger.sql event
|
|
assert_match([[pk.name, 10]].inspect, logger.debugs.first)
|
|
end
|
|
end
|
|
end
|
|
end
|