mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
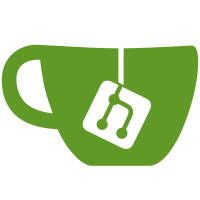
We sometimes say "✂️ newline after `private`" in a code review (e.g. https://github.com/rails/rails/pull/18546#discussion_r23188776, https://github.com/rails/rails/pull/34832#discussion_r244847195). Now `Layout/EmptyLinesAroundAccessModifier` cop have new enforced style `EnforcedStyle: only_before` (https://github.com/rubocop-hq/rubocop/pull/7059). That cop and enforced style will reduce the our code review cost.
46 lines
1.3 KiB
Ruby
46 lines
1.3 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
module ActionDispatch
|
|
# This is a class that abstracts away an asserted response. It purposely
|
|
# does not inherit from Response because it doesn't need it. That means it
|
|
# does not have headers or a body.
|
|
class AssertionResponse
|
|
attr_reader :code, :name
|
|
|
|
GENERIC_RESPONSE_CODES = { # :nodoc:
|
|
success: "2XX",
|
|
missing: "404",
|
|
redirect: "3XX",
|
|
error: "5XX"
|
|
}
|
|
|
|
# Accepts a specific response status code as an Integer (404) or String
|
|
# ('404') or a response status range as a Symbol pseudo-code (:success,
|
|
# indicating any 200-299 status code).
|
|
def initialize(code_or_name)
|
|
if code_or_name.is_a?(Symbol)
|
|
@name = code_or_name
|
|
@code = code_from_name(code_or_name)
|
|
else
|
|
@name = name_from_code(code_or_name)
|
|
@code = code_or_name
|
|
end
|
|
|
|
raise ArgumentError, "Invalid response name: #{name}" if @code.nil?
|
|
raise ArgumentError, "Invalid response code: #{code}" if @name.nil?
|
|
end
|
|
|
|
def code_and_name
|
|
"#{code}: #{name}"
|
|
end
|
|
|
|
private
|
|
def code_from_name(name)
|
|
GENERIC_RESPONSE_CODES[name] || Rack::Utils::SYMBOL_TO_STATUS_CODE[name]
|
|
end
|
|
|
|
def name_from_code(code)
|
|
GENERIC_RESPONSE_CODES.invert[code] || Rack::Utils::HTTP_STATUS_CODES[code]
|
|
end
|
|
end
|
|
end
|