mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
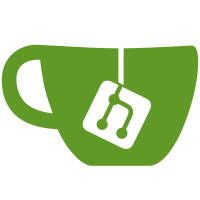
We sometimes say "✂️ newline after `private`" in a code review (e.g. https://github.com/rails/rails/pull/18546#discussion_r23188776, https://github.com/rails/rails/pull/34832#discussion_r244847195). Now `Layout/EmptyLinesAroundAccessModifier` cop have new enforced style `EnforcedStyle: only_before` (https://github.com/rubocop-hq/rubocop/pull/7059). That cop and enforced style will reduce the our code review cost.
62 lines
1.4 KiB
Ruby
62 lines
1.4 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "active_support"
|
|
require "active_support/testing/autorun"
|
|
require "rails/configuration"
|
|
require "active_support/test_case"
|
|
require "minitest/mock"
|
|
|
|
module Rails
|
|
module Configuration
|
|
class MiddlewareStackProxyTest < ActiveSupport::TestCase
|
|
def setup
|
|
@stack = MiddlewareStackProxy.new
|
|
end
|
|
|
|
def test_playback_insert_before
|
|
@stack.insert_before :foo
|
|
assert_playback :insert_before, :foo
|
|
end
|
|
|
|
def test_playback_insert_after
|
|
@stack.insert_after :foo
|
|
assert_playback :insert_after, :foo
|
|
end
|
|
|
|
def test_playback_swap
|
|
@stack.swap :foo
|
|
assert_playback :swap, :foo
|
|
end
|
|
|
|
def test_playback_use
|
|
@stack.use :foo
|
|
assert_playback :use, :foo
|
|
end
|
|
|
|
def test_playback_delete
|
|
@stack.delete :foo
|
|
assert_playback :delete, :foo
|
|
end
|
|
|
|
def test_order
|
|
@stack.swap :foo
|
|
@stack.delete :foo
|
|
|
|
mock = Minitest::Mock.new
|
|
mock.expect :send, nil, [:swap, :foo]
|
|
mock.expect :send, nil, [:delete, :foo]
|
|
|
|
@stack.merge_into mock
|
|
mock.verify
|
|
end
|
|
|
|
private
|
|
def assert_playback(msg_name, args)
|
|
mock = Minitest::Mock.new
|
|
mock.expect :send, nil, [msg_name, args]
|
|
@stack.merge_into(mock)
|
|
mock.verify
|
|
end
|
|
end
|
|
end
|
|
end
|