mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
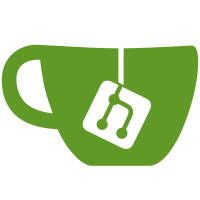
* request.formats is much simpler now * For XHRs or Accept headers with a single item, we use the Accept header * For other requests, we use params[:format] or fallback to HTML * This is primarily to work around the fact that browsers provide completely broken Accept headers, so we have to whitelist the few cases we can specifically isolate and treat other requests as coming from the browser * For APIs, we can support single-item Accept headers, which disambiguates from the browsers * Requests to an action that only has an XML template from the browser will no longer find the template. This worked previously because most browsers provide a catch-all */*, but this was mostly accidental behavior. If you want to serve XML, either use the :xml format in links, or explicitly specify the XML template: render "template.xml".
115 lines
4.7 KiB
Ruby
115 lines
4.7 KiB
Ruby
require 'abstract_unit'
|
|
|
|
class JavaScriptHelperTest < ActionView::TestCase
|
|
tests ActionView::Helpers::JavaScriptHelper
|
|
|
|
def _evaluate_assigns_and_ivars() end
|
|
|
|
attr_accessor :formats, :output_buffer
|
|
|
|
def reset_formats(format)
|
|
@format = format
|
|
end
|
|
|
|
def setup
|
|
super
|
|
@template = self
|
|
end
|
|
|
|
def _evaluate_assigns_and_ivars() end
|
|
|
|
def test_escape_javascript
|
|
assert_equal '', escape_javascript(nil)
|
|
assert_equal %(This \\"thing\\" is really\\n netos\\'), escape_javascript(%(This "thing" is really\n netos'))
|
|
assert_equal %(backslash\\\\test), escape_javascript( %(backslash\\test) )
|
|
assert_equal %(dont <\\/close> tags), escape_javascript(%(dont </close> tags))
|
|
end
|
|
|
|
def test_link_to_function
|
|
assert_dom_equal %(<a href="#" onclick="alert('Hello world!'); return false;">Greeting</a>),
|
|
link_to_function("Greeting", "alert('Hello world!')")
|
|
end
|
|
|
|
def test_link_to_function_with_existing_onclick
|
|
assert_dom_equal %(<a href="#" onclick="confirm('Sanity!'); alert('Hello world!'); return false;">Greeting</a>),
|
|
link_to_function("Greeting", "alert('Hello world!')", :onclick => "confirm('Sanity!')")
|
|
end
|
|
|
|
def test_link_to_function_with_rjs_block
|
|
html = link_to_function( "Greet me!" ) do |page|
|
|
page.replace_html 'header', "<h1>Greetings</h1>"
|
|
end
|
|
assert_dom_equal %(<a href="#" onclick="Element.update("header", "\\u003Ch1\\u003EGreetings\\u003C/h1\\u003E");; return false;">Greet me!</a>), html
|
|
end
|
|
|
|
def test_link_to_function_with_rjs_block_and_options
|
|
html = link_to_function( "Greet me!", :class => "updater" ) do |page|
|
|
page.replace_html 'header', "<h1>Greetings</h1>"
|
|
end
|
|
assert_dom_equal %(<a href="#" class="updater" onclick="Element.update("header", "\\u003Ch1\\u003EGreetings\\u003C/h1\\u003E");; return false;">Greet me!</a>), html
|
|
end
|
|
|
|
def test_link_to_function_with_href
|
|
assert_dom_equal %(<a href="http://example.com/" onclick="alert('Hello world!'); return false;">Greeting</a>),
|
|
link_to_function("Greeting", "alert('Hello world!')", :href => 'http://example.com/')
|
|
end
|
|
|
|
def test_button_to_function
|
|
assert_dom_equal %(<input type="button" onclick="alert('Hello world!');" value="Greeting" />),
|
|
button_to_function("Greeting", "alert('Hello world!')")
|
|
end
|
|
|
|
def test_button_to_function_with_rjs_block
|
|
html = button_to_function( "Greet me!" ) do |page|
|
|
page.replace_html 'header', "<h1>Greetings</h1>"
|
|
end
|
|
assert_dom_equal %(<input type="button" onclick="Element.update("header", "\\u003Ch1\\u003EGreetings\\u003C/h1\\u003E");;" value="Greet me!" />), html
|
|
end
|
|
|
|
def test_button_to_function_with_rjs_block_and_options
|
|
html = button_to_function( "Greet me!", :class => "greeter" ) do |page|
|
|
page.replace_html 'header', "<h1>Greetings</h1>"
|
|
end
|
|
assert_dom_equal %(<input type="button" class="greeter" onclick="Element.update("header", "\\u003Ch1\\u003EGreetings\\u003C\/h1\\u003E");;" value="Greet me!" />), html
|
|
end
|
|
|
|
def test_button_to_function_with_onclick
|
|
assert_dom_equal "<input onclick=\"alert('Goodbye World :('); alert('Hello world!');\" type=\"button\" value=\"Greeting\" />",
|
|
button_to_function("Greeting", "alert('Hello world!')", :onclick => "alert('Goodbye World :(')")
|
|
end
|
|
|
|
def test_button_to_function_without_function
|
|
assert_dom_equal "<input onclick=\";\" type=\"button\" value=\"Greeting\" />",
|
|
button_to_function("Greeting")
|
|
end
|
|
|
|
def test_javascript_tag
|
|
self.output_buffer = 'foo'
|
|
|
|
assert_dom_equal "<script type=\"text/javascript\">\n//<![CDATA[\nalert('hello')\n//]]>\n</script>",
|
|
javascript_tag("alert('hello')")
|
|
|
|
assert_equal 'foo', output_buffer, 'javascript_tag without a block should not concat to output_buffer'
|
|
end
|
|
|
|
def test_javascript_tag_with_options
|
|
assert_dom_equal "<script id=\"the_js_tag\" type=\"text/javascript\">\n//<![CDATA[\nalert('hello')\n//]]>\n</script>",
|
|
javascript_tag("alert('hello')", :id => "the_js_tag")
|
|
end
|
|
|
|
def test_javascript_tag_with_block_in_erb
|
|
__in_erb_template = ''
|
|
javascript_tag { concat "alert('hello')" }
|
|
assert_dom_equal "<script type=\"text/javascript\">\n//<![CDATA[\nalert('hello')\n//]]>\n</script>", output_buffer
|
|
end
|
|
|
|
def test_javascript_tag_with_block_and_options_in_erb
|
|
__in_erb_template = ''
|
|
javascript_tag(:id => "the_js_tag") { concat "alert('hello')" }
|
|
assert_dom_equal "<script id=\"the_js_tag\" type=\"text/javascript\">\n//<![CDATA[\nalert('hello')\n//]]>\n</script>", output_buffer
|
|
end
|
|
|
|
def test_javascript_cdata_section
|
|
assert_dom_equal "\n//<![CDATA[\nalert('hello')\n//]]>\n", javascript_cdata_section("alert('hello')")
|
|
end
|
|
end
|