mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
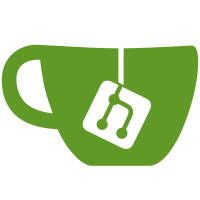
This commit fixes `deprecate` so that it preserves method visibility (like it
did previously when it was utilizing `alias_method_chain`).
When Module#prepend replaced alias_method_chain in a982a42
it caused deprecated
methods to always become public.
`alias_method_chain` had this bit of code:
https://github.com/rails/rails/blob/v5.0.6/activesupport/lib/active_support/core_ext/module/aliasing.rb#L40-L47
which preserved method visibility.
Without this fix, a workaround would be:
```ruby
class C8
private
def new_method
end
def old_method
end
deprecate :old_method, :new_method
# workaround:
instance_method(:old_method).owner.send(:private, :old_method)
end
```
Because the visibility needs to be fixed on the Module prepended by
MethodWrapper.
58 lines
2.1 KiB
Ruby
58 lines
2.1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "abstract_unit"
|
|
require "active_support/deprecation"
|
|
|
|
class MethodWrappersTest < ActiveSupport::TestCase
|
|
def setup
|
|
@klass = Class.new do
|
|
def new_method; "abc" end
|
|
alias_method :old_method, :new_method
|
|
|
|
protected
|
|
|
|
def new_protected_method; "abc" end
|
|
alias_method :old_protected_method, :new_protected_method
|
|
|
|
private
|
|
|
|
def new_private_method; "abc" end
|
|
alias_method :old_private_method, :new_private_method
|
|
end
|
|
end
|
|
|
|
def test_deprecate_methods_warning_default
|
|
warning = /old_method is deprecated and will be removed from Rails \d.\d \(use new_method instead\)/
|
|
ActiveSupport::Deprecation.deprecate_methods(@klass, old_method: :new_method)
|
|
|
|
assert_deprecated(warning) { assert_equal "abc", @klass.new.old_method }
|
|
end
|
|
|
|
def test_deprecate_methods_warning_with_optional_deprecator
|
|
warning = /old_method is deprecated and will be removed from MyGem next-release \(use new_method instead\)/
|
|
deprecator = ActiveSupport::Deprecation.new("next-release", "MyGem")
|
|
ActiveSupport::Deprecation.deprecate_methods(@klass, old_method: :new_method, deprecator: deprecator)
|
|
|
|
assert_deprecated(warning, deprecator) { assert_equal "abc", @klass.new.old_method }
|
|
end
|
|
|
|
def test_deprecate_methods_warning_when_deprecated_with_custom_deprecator
|
|
warning = /old_method is deprecated and will be removed from MyGem next-release \(use new_method instead\)/
|
|
deprecator = ActiveSupport::Deprecation.new("next-release", "MyGem")
|
|
deprecator.deprecate_methods(@klass, old_method: :new_method)
|
|
|
|
assert_deprecated(warning, deprecator) { assert_equal "abc", @klass.new.old_method }
|
|
end
|
|
|
|
def test_deprecate_methods_protected_method
|
|
ActiveSupport::Deprecation.deprecate_methods(@klass, old_protected_method: :new_protected_method)
|
|
|
|
assert(@klass.protected_method_defined?(:old_protected_method))
|
|
end
|
|
|
|
def test_deprecate_methods_private_method
|
|
ActiveSupport::Deprecation.deprecate_methods(@klass, old_private_method: :new_private_method)
|
|
|
|
assert(@klass.private_method_defined?(:old_private_method))
|
|
end
|
|
end
|