mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
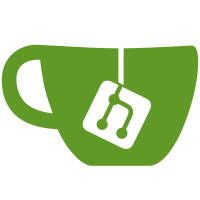
* Move #set_cookie and #delete_cookie inline to optimize. These optimizations should almost certainly be sent back upstream to Rack. The optimization involves using an ivar for cookies instead of indexing into the headers each time. * Was able to use a bare Hash for headers now that cookies have their own joining semantics (some code assumed that the raw cookies were an Array). * Cache blankness of body on body= * Improve expand_cache_key for Arrays of a single element (common in our case) * Use a simple layout condition check unless conditions are used * Cache visible actions * Lazily load the UrlRewriter * Make etag an ivar that is set on prepare!
88 lines
2.2 KiB
Ruby
88 lines
2.2 KiB
Ruby
require 'active_support/test_case'
|
|
|
|
module ActionView
|
|
class Base
|
|
alias_method :initialize_without_template_tracking, :initialize
|
|
def initialize(*args)
|
|
@_rendered = { :template => nil, :partials => Hash.new(0) }
|
|
initialize_without_template_tracking(*args)
|
|
end
|
|
|
|
attr_internal :rendered
|
|
end
|
|
|
|
class Template
|
|
alias_method :render_without_tracking, :render
|
|
def render(view, locals, &blk)
|
|
rendered = view.rendered
|
|
rendered[:partials][self] += 1 if partial?
|
|
rendered[:template] ||= []
|
|
rendered[:template] << self
|
|
render_without_tracking(view, locals, &blk)
|
|
end
|
|
end
|
|
|
|
class TestCase < ActiveSupport::TestCase
|
|
include ActionDispatch::Assertions
|
|
include ActionController::TestProcess
|
|
include ActionView::Context
|
|
|
|
class_inheritable_accessor :helper_class
|
|
@@helper_class = nil
|
|
|
|
class << self
|
|
def tests(helper_class)
|
|
self.helper_class = helper_class
|
|
end
|
|
|
|
def helper_class
|
|
if current_helper_class = read_inheritable_attribute(:helper_class)
|
|
current_helper_class
|
|
else
|
|
self.helper_class = determine_default_helper_class(name)
|
|
end
|
|
end
|
|
|
|
def determine_default_helper_class(name)
|
|
name.sub(/Test$/, '').constantize
|
|
rescue NameError
|
|
nil
|
|
end
|
|
end
|
|
|
|
include ActionView::Helpers
|
|
include ActionController::PolymorphicRoutes
|
|
include ActionController::RecordIdentifier
|
|
|
|
setup :setup_with_helper_class
|
|
|
|
def setup_with_helper_class
|
|
if helper_class && !self.class.ancestors.include?(helper_class)
|
|
self.class.send(:include, helper_class)
|
|
end
|
|
|
|
self.output_buffer = ''
|
|
end
|
|
|
|
class TestController < ActionController::Base
|
|
attr_accessor :request, :response, :params
|
|
|
|
def initialize
|
|
@request = ActionController::TestRequest.new
|
|
@response = ActionController::TestResponse.new
|
|
|
|
@params = {}
|
|
end
|
|
end
|
|
|
|
protected
|
|
attr_accessor :output_buffer
|
|
|
|
private
|
|
def method_missing(selector, *args)
|
|
controller = TestController.new
|
|
return controller.__send__(selector, *args) if ActionController::Routing::Routes.named_routes.helpers.include?(selector)
|
|
super
|
|
end
|
|
end
|
|
end
|