mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
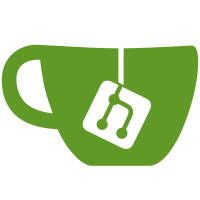
- I made a change in 0d3aec4969
to output a log if a job was aborted
in a before callbacks. I didn't take in consideration that a job
could return a falsy value and thus it would wrongly log
that the job was aborted.
This fixes the problem by checking if the callback chain was halted
rather than the return value of the job.
37 lines
1,012 B
Ruby
37 lines
1,012 B
Ruby
# frozen_string_literal: true
|
|
|
|
module ActiveJob
|
|
module Instrumentation #:nodoc:
|
|
extend ActiveSupport::Concern
|
|
|
|
included do
|
|
around_enqueue do |_, block|
|
|
scheduled_at ? instrument(:enqueue_at, &block) : instrument(:enqueue, &block)
|
|
end
|
|
|
|
around_perform do |_, block|
|
|
instrument :perform_start
|
|
instrument :perform, &block
|
|
end
|
|
end
|
|
|
|
private
|
|
def instrument(operation, payload = {}, &block)
|
|
enhanced_block = ->(event_payload) do
|
|
block.call if block
|
|
if defined?(@_halted_callback_hook_called) && @_halted_callback_hook_called
|
|
event_payload[:aborted] = true
|
|
@_halted_callback_hook_called = nil
|
|
end
|
|
end
|
|
|
|
ActiveSupport::Notifications.instrument \
|
|
"#{operation}.active_job", payload.merge(adapter: queue_adapter, job: self), &enhanced_block
|
|
end
|
|
|
|
def halted_callback_hook(_)
|
|
super
|
|
@_halted_callback_hook_called = true
|
|
end
|
|
end
|
|
end
|