mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
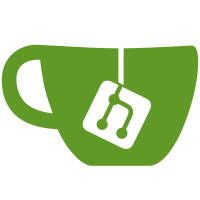
MySQL supports DELETE with LIMIT and ORDER BY. https://dev.mysql.com/doc/refman/8.0/en/delete.html Before: ``` Post Destroy (1.0ms) DELETE FROM `posts` WHERE `posts`.`id` IN (SELECT `id` FROM (SELECT `posts`.`id` FROM `posts` WHERE `posts`.`author_id` = ? ORDER BY `posts`.`id` ASC LIMIT ?) __active_record_temp) [["author_id", 1], ["LIMIT", 1]] ``` After: ``` Post Destroy (0.4ms) DELETE FROM `posts` WHERE `posts`.`author_id` = ? ORDER BY `posts`.`id` ASC LIMIT ? [["author_id", 1], ["LIMIT", 1]] ```
34 lines
640 B
Ruby
34 lines
640 B
Ruby
# frozen_string_literal: true
|
|
|
|
module Arel # :nodoc: all
|
|
class UpdateManager < Arel::TreeManager
|
|
include TreeManager::StatementMethods
|
|
|
|
def initialize
|
|
super
|
|
@ast = Nodes::UpdateStatement.new
|
|
@ctx = @ast
|
|
end
|
|
|
|
###
|
|
# UPDATE +table+
|
|
def table(table)
|
|
@ast.relation = table
|
|
self
|
|
end
|
|
|
|
def set(values)
|
|
if String === values
|
|
@ast.values = [values]
|
|
else
|
|
@ast.values = values.map { |column, value|
|
|
Nodes::Assignment.new(
|
|
Nodes::UnqualifiedColumn.new(column),
|
|
value
|
|
)
|
|
}
|
|
end
|
|
self
|
|
end
|
|
end
|
|
end
|