mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
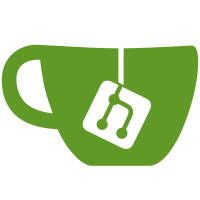
If you run a generator such as: ``` rails generate model accounts supplier:references ``` The resulting migration will now add the corresponding foreign key constraint unless the reference was specified to be polymorphic.
286 lines
11 KiB
Ruby
286 lines
11 KiB
Ruby
require 'generators/generators_test_helper'
|
|
require 'rails/generators/rails/migration/migration_generator'
|
|
|
|
class MigrationGeneratorTest < Rails::Generators::TestCase
|
|
include GeneratorsTestHelper
|
|
|
|
def test_migration
|
|
migration = "change_title_body_from_posts"
|
|
run_generator [migration]
|
|
assert_migration "db/migrate/#{migration}.rb", /class ChangeTitleBodyFromPosts < ActiveRecord::Migration/
|
|
end
|
|
|
|
def test_migrations_generated_simultaneously
|
|
migrations = ["change_title_body_from_posts", "change_email_from_comments"]
|
|
|
|
first_migration_number, second_migration_number = migrations.collect do |migration|
|
|
run_generator [migration]
|
|
file_name = migration_file_name "db/migrate/#{migration}.rb"
|
|
|
|
File.basename(file_name).split('_').first
|
|
end
|
|
|
|
assert_not_equal first_migration_number, second_migration_number
|
|
end
|
|
|
|
def test_migration_with_class_name
|
|
migration = "ChangeTitleBodyFromPosts"
|
|
run_generator [migration]
|
|
assert_migration "db/migrate/change_title_body_from_posts.rb", /class #{migration} < ActiveRecord::Migration/
|
|
end
|
|
|
|
def test_migration_with_invalid_file_name
|
|
migration = "add_something:datetime"
|
|
assert_raise ActiveRecord::IllegalMigrationNameError do
|
|
run_generator [migration]
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_attributes
|
|
migration = "add_title_body_to_posts"
|
|
run_generator [migration, "title:string", "body:text"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_column :posts, :title, :string/, change)
|
|
assert_match(/add_column :posts, :body, :text/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_remove_migration_with_indexed_attribute
|
|
migration = "remove_title_body_from_posts"
|
|
run_generator [migration, "title:string:index", "body:text"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/remove_column :posts, :title, :string/, change)
|
|
assert_match(/remove_column :posts, :body, :text/, change)
|
|
assert_match(/remove_index :posts, :title/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_remove_migration_with_attributes
|
|
migration = "remove_title_body_from_posts"
|
|
run_generator [migration, "title:string", "body:text"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/remove_column :posts, :title, :string/, change)
|
|
assert_match(/remove_column :posts, :body, :text/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_remove_migration_with_references_options
|
|
migration = "remove_references_from_books"
|
|
run_generator [migration, "author:belongs_to", "distributor:references{polymorphic}"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/remove_reference :books, :author, index: true/, change)
|
|
assert_match(/remove_reference :books, :distributor, polymorphic: true, index: true/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_remove_migration_with_references_removes_foreign_keys
|
|
migration = "remove_references_from_books"
|
|
run_generator [migration, "author:belongs_to", "distributor:references{polymorphic}"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/remove_foreign_key :books, :authors/, change)
|
|
assert_no_match(/remove_foreign_key :books, :distributors/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_attributes_and_indices
|
|
migration = "add_title_with_index_and_body_to_posts"
|
|
run_generator [migration, "title:string:index", "body:text", "user_id:integer:uniq"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_column :posts, :title, :string/, change)
|
|
assert_match(/add_column :posts, :body, :text/, change)
|
|
assert_match(/add_column :posts, :user_id, :integer/, change)
|
|
assert_match(/add_index :posts, :title/, change)
|
|
assert_match(/add_index :posts, :user_id, unique: true/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_attributes_and_wrong_index_declaration
|
|
migration = "add_title_and_content_to_books"
|
|
run_generator [migration, "title:string:inex", "content:text", "user_id:integer:unik"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_column :books, :title, :string/, change)
|
|
assert_match(/add_column :books, :content, :text/, change)
|
|
assert_match(/add_column :books, :user_id, :integer/, change)
|
|
end
|
|
assert_no_match(/add_index :books, :title/, content)
|
|
assert_no_match(/add_index :books, :user_id/, content)
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_attributes_without_type_and_index
|
|
migration = "add_title_with_index_and_body_to_posts"
|
|
run_generator [migration, "title:index", "body:text", "user_uuid:uniq"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_column :posts, :title, :string/, change)
|
|
assert_match(/add_column :posts, :body, :text/, change)
|
|
assert_match(/add_column :posts, :user_uuid, :string/, change)
|
|
assert_match(/add_index :posts, :title/, change)
|
|
assert_match(/add_index :posts, :user_uuid, unique: true/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_attributes_index_declaration_and_attribute_options
|
|
migration = "add_title_and_content_to_books"
|
|
run_generator [migration, "title:string{40}:index", "content:string{255}", "price:decimal{1,2}:index", "discount:decimal{3.4}:uniq"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_column :books, :title, :string, limit: 40/, change)
|
|
assert_match(/add_column :books, :content, :string, limit: 255/, change)
|
|
assert_match(/add_column :books, :price, :decimal, precision: 1, scale: 2/, change)
|
|
assert_match(/add_column :books, :discount, :decimal, precision: 3, scale: 4/, change)
|
|
end
|
|
assert_match(/add_index :books, :title/, content)
|
|
assert_match(/add_index :books, :price/, content)
|
|
assert_match(/add_index :books, :discount, unique: true/, content)
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_references_options
|
|
migration = "add_references_to_books"
|
|
run_generator [migration, "author:belongs_to", "distributor:references{polymorphic}"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_reference :books, :author, index: true/, change)
|
|
assert_match(/add_reference :books, :distributor, polymorphic: true, index: true/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_required_references
|
|
migration = "add_references_to_books"
|
|
run_generator [migration, "author:belongs_to{required}", "distributor:references{polymorphic,required}"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_reference :books, :author, index: true, null: false/, change)
|
|
assert_match(/add_reference :books, :distributor, polymorphic: true, index: true, null: false/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_add_migration_with_references_adds_foreign_keys
|
|
migration = "add_references_to_books"
|
|
run_generator [migration, "author:belongs_to", "distributor:references{polymorphic}"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_foreign_key :books, :authors/, change)
|
|
assert_no_match(/add_foreign_key :books, :distributors/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_create_join_table_migration
|
|
migration = "add_media_join_table"
|
|
run_generator [migration, "artist_id", "musics:uniq"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/create_join_table :artists, :musics/, change)
|
|
assert_match(/# t.index \[:artist_id, :music_id\]/, change)
|
|
assert_match(/ t.index \[:music_id, :artist_id\], unique: true/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_create_table_migration
|
|
run_generator ["create_books", "title:string", "content:text"]
|
|
assert_migration "db/migrate/create_books.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/create_table :books/, change)
|
|
assert_match(/ t\.string :title/, change)
|
|
assert_match(/ t\.text :content/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_should_create_empty_migrations_if_name_not_start_with_add_or_remove_or_create
|
|
migration = "delete_books"
|
|
run_generator [migration, "title:string", "content:text"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/^\s*$/, change)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_properly_identifies_usage_file
|
|
assert generator_class.send(:usage_path)
|
|
end
|
|
|
|
def test_migration_with_singular_table_name
|
|
with_singular_table_name do
|
|
migration = "add_title_body_to_post"
|
|
run_generator [migration, 'title:string']
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/add_column :post, :title, :string/, change)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_create_join_table_migration_with_singular_table_name
|
|
with_singular_table_name do
|
|
migration = "add_media_join_table"
|
|
run_generator [migration, "artist_id", "music:uniq"]
|
|
|
|
assert_migration "db/migrate/#{migration}.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/create_join_table :artist, :music/, change)
|
|
assert_match(/# t.index \[:artist_id, :music_id\]/, change)
|
|
assert_match(/ t.index \[:music_id, :artist_id\], unique: true/, change)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_create_table_migration_with_singular_table_name
|
|
with_singular_table_name do
|
|
run_generator ["create_book", "title:string", "content:text"]
|
|
assert_migration "db/migrate/create_book.rb" do |content|
|
|
assert_method :change, content do |change|
|
|
assert_match(/create_table :book/, change)
|
|
assert_match(/ t\.string :title/, change)
|
|
assert_match(/ t\.text :content/, change)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
private
|
|
|
|
def with_singular_table_name
|
|
old_state = ActiveRecord::Base.pluralize_table_names
|
|
ActiveRecord::Base.pluralize_table_names = false
|
|
yield
|
|
ensure
|
|
ActiveRecord::Base.pluralize_table_names = old_state
|
|
end
|
|
end
|