mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
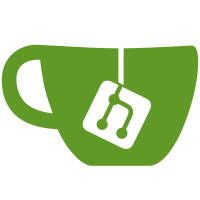
In the end I think the pain of implementing this seamlessly was not worth the gain provided. The intention was that it would allow plain ruby objects that might not live in your main application to be subclassed and have persistence mixed in. But I've decided that the benefit of doing that is not worth the amount of complexity that the implementation introduced.
67 lines
1.4 KiB
Ruby
67 lines
1.4 KiB
Ruby
module ActiveRecord
|
|
###
|
|
# This class encapsulates a Result returned from calling +exec_query+ on any
|
|
# database connection adapter. For example:
|
|
#
|
|
# x = ActiveRecord::Base.connection.exec_query('SELECT * FROM foo')
|
|
# x # => #<ActiveRecord::Result:0xdeadbeef>
|
|
class Result
|
|
include Enumerable
|
|
|
|
attr_reader :columns, :rows, :column_types
|
|
|
|
def initialize(columns, rows, column_types = {})
|
|
@columns = columns
|
|
@rows = rows
|
|
@hash_rows = nil
|
|
@column_types = column_types
|
|
end
|
|
|
|
def each
|
|
hash_rows.each { |row| yield row }
|
|
end
|
|
|
|
def to_hash
|
|
hash_rows
|
|
end
|
|
|
|
alias :map! :map
|
|
alias :collect! :map
|
|
|
|
# Returns true if there are no records.
|
|
def empty?
|
|
rows.empty?
|
|
end
|
|
|
|
def to_ary
|
|
hash_rows
|
|
end
|
|
|
|
def [](idx)
|
|
hash_rows[idx]
|
|
end
|
|
|
|
def last
|
|
hash_rows.last
|
|
end
|
|
|
|
def initialize_copy(other)
|
|
@columns = columns.dup
|
|
@rows = rows.dup
|
|
@hash_rows = nil
|
|
end
|
|
|
|
private
|
|
def hash_rows
|
|
@hash_rows ||=
|
|
begin
|
|
# We freeze the strings to prevent them getting duped when
|
|
# used as keys in ActiveRecord::Base's @attributes hash
|
|
columns = @columns.map { |c| c.dup.freeze }
|
|
@rows.map { |row|
|
|
Hash[columns.zip(row)]
|
|
}
|
|
end
|
|
end
|
|
end
|
|
end
|