mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
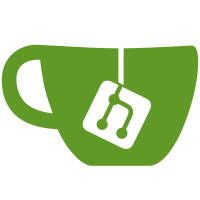
The delegation methods to named scope are defined when `method_missing` is invoked on the relation. Since #29301, the receiver in the named scope is changed to the relation like others (e.g. `default_scope`, etc) for consistency. Most named scopes would be delegated from relation by `method_missing`, since we don't allow scopes to be defined which conflict with instance methods on `Relation` (#31179). But if a named scope is defined with the same name as any method on the `superclass` (e.g. `Kernel.open`), the `method_missing` on the relation is not invoked. To address the issue, make the delegation methods to named scope is generated in the definition time. Fixes #34098.
41 lines
1 KiB
Ruby
41 lines
1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
class Account < ActiveRecord::Base
|
|
belongs_to :firm, class_name: "Company"
|
|
belongs_to :unautosaved_firm, foreign_key: "firm_id", class_name: "Firm", autosave: false
|
|
|
|
alias_attribute :available_credit, :credit_limit
|
|
|
|
def self.destroyed_account_ids
|
|
@destroyed_account_ids ||= Hash.new { |h, k| h[k] = [] }
|
|
end
|
|
|
|
# Test private kernel method through collection proxy using has_many.
|
|
scope :open, -> { where("firm_name = ?", "37signals") }
|
|
scope :available, -> { open }
|
|
|
|
before_destroy do |account|
|
|
if account.firm
|
|
Account.destroyed_account_ids[account.firm.id] << account.id
|
|
end
|
|
end
|
|
|
|
validate :check_empty_credit_limit
|
|
|
|
private
|
|
def check_empty_credit_limit
|
|
errors.add("credit_limit", :blank) if credit_limit.blank?
|
|
end
|
|
|
|
def private_method
|
|
"Sir, yes sir!"
|
|
end
|
|
end
|
|
|
|
class SubAccount < Account
|
|
def self.instantiate_instance_of(klass, attributes, column_types = {}, &block)
|
|
klass = superclass
|
|
super
|
|
end
|
|
private_class_method :instantiate_instance_of
|
|
end
|