mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
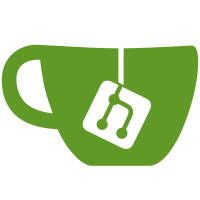
Sometimes when we are preloading associations on records, we already have the association objects loaded in-memory. But the Preloader will go to the database to fetch them anyways because there is no way to tell it about these loaded objects. This PR adds a new `available_records` argument to supply the Preloader with a set of in-memory records that it can use to fill associations without making a database query. `available_records` is an array of ActiveRecord::Base objects. Mixed models are supported. Preloader will use these records to try to fill the requested associations before querying the database. This can save database queries by reusing in-memory objects. The optimization is only applied to single associations (i.e. :belongs_to, :has_one) as we must query the database to do an exhaustive lookup for collections (i.e. :has_many). It also requires the query to have no scopes as scopes can filter associations even if they are already in memory. ```ruby comment = Comment.last post = Post.find_by(id: comment.post_id) all_authors = Author.all.to_a Preloader.new([comment], [:post, :author]).call Preloader.new([comment], [:post, :author], available_records: [post, all_authors]).call ``` Co-Authored-By: John Hawthorn <john@hawthorn.email>
8 lines
249 B
Ruby
8 lines
249 B
Ruby
# frozen_string_literal: true
|
|
|
|
class Essay < ActiveRecord::Base
|
|
belongs_to :author, primary_key: :name
|
|
belongs_to :writer, primary_key: :name, polymorphic: true
|
|
belongs_to :category, primary_key: :name
|
|
has_one :owner, primary_key: :name
|
|
end
|