mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
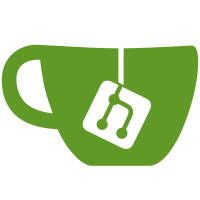
Email does not support relative links since there is no implicit host. Therefore all links inside of emails must be fully qualified URLs. All path helpers are now deprecated. When removed, the error will give early indication to developers to use `*_url` methods instead. Currently if a developer uses a `*_path` helper, their tests and `mail_view` will not catch the mistake. The only way to see the error is by sending emails in production. Preventing sending out emails with non-working path's is the desired end goal of this PR. Currently path helpers are mixed-in to controllers (the ActionMailer::Base acts as a controller). All `*_url` and `*_path` helpers are made available through the same module. This PR separates this behavior into two modules so we can extend the `*_path` methods to add a Deprecation to them. Once deprecated we can use this same area to raise a NoMethodError and add an informative message directing the developer to use `*_url` instead. The module with warnings is only mixed in when a controller returns false from the newly added `supports_relative_path?`. Paired @sgrif & @schneems
494 lines
13 KiB
Ruby
494 lines
13 KiB
Ruby
require 'isolation/abstract_unit'
|
|
require 'rack/test'
|
|
module ApplicationTests
|
|
class MailerPreviewsTest < ActiveSupport::TestCase
|
|
include ActiveSupport::Testing::Isolation
|
|
include Rack::Test::Methods
|
|
|
|
def setup
|
|
build_app
|
|
boot_rails
|
|
end
|
|
|
|
def teardown
|
|
teardown_app
|
|
end
|
|
|
|
test "/rails/mailers is accessible in development" do
|
|
app("development")
|
|
get "/rails/mailers"
|
|
assert_equal 200, last_response.status
|
|
end
|
|
|
|
test "/rails/mailers is not accessible in production" do
|
|
app("production")
|
|
get "/rails/mailers"
|
|
assert_equal 404, last_response.status
|
|
end
|
|
|
|
test "/rails/mailers is accessible with correct configuraiton" do
|
|
add_to_config "config.action_mailer.show_previews = true"
|
|
app("production")
|
|
get "/rails/mailers"
|
|
assert_equal 200, last_response.status
|
|
end
|
|
|
|
test "/rails/mailers is not accessible with show_previews = false" do
|
|
add_to_config "config.action_mailer.show_previews = false"
|
|
app("development")
|
|
get "/rails/mailers"
|
|
assert_equal 404, last_response.status
|
|
end
|
|
|
|
test "mailer previews are loaded from the default preview_path" do
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
assert_match '<li><a href="/rails/mailers/notifier/foo">foo</a></li>', last_response.body
|
|
end
|
|
|
|
test "mailer previews are loaded from a custom preview_path" do
|
|
add_to_config "config.action_mailer.preview_path = '#{app_path}/lib/mailer_previews'"
|
|
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
app_file 'lib/mailer_previews/notifier_preview.rb', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
assert_match '<li><a href="/rails/mailers/notifier/foo">foo</a></li>', last_response.body
|
|
end
|
|
|
|
test "mailer previews are reloaded across requests" do
|
|
app('development')
|
|
|
|
get "/rails/mailers"
|
|
assert_no_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
|
|
remove_file 'test/mailers/previews/notifier_preview.rb'
|
|
sleep(1)
|
|
|
|
get "/rails/mailers"
|
|
assert_no_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
end
|
|
|
|
test "mailer preview actions are added and removed" do
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
assert_match '<li><a href="/rails/mailers/notifier/foo">foo</a></li>', last_response.body
|
|
assert_no_match '<li><a href="/rails/mailers/notifier/bar">bar</a></li>', last_response.body
|
|
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
|
|
def bar
|
|
mail to: "to@example.net"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
text_template 'notifier/bar', <<-RUBY
|
|
Goodbye, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
|
|
def bar
|
|
Notifier.bar
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
sleep(1)
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
assert_match '<li><a href="/rails/mailers/notifier/foo">foo</a></li>', last_response.body
|
|
assert_match '<li><a href="/rails/mailers/notifier/bar">bar</a></li>', last_response.body
|
|
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
remove_file 'app/views/notifier/bar.text.erb'
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
sleep(1)
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
assert_match '<li><a href="/rails/mailers/notifier/foo">foo</a></li>', last_response.body
|
|
assert_no_match '<li><a href="/rails/mailers/notifier/bar">bar</a></li>', last_response.body
|
|
end
|
|
|
|
test "mailer previews are reloaded from a custom preview_path" do
|
|
add_to_config "config.action_mailer.preview_path = '#{app_path}/lib/mailer_previews'"
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers"
|
|
assert_no_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
app_file 'lib/mailer_previews/notifier_preview.rb', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
get "/rails/mailers"
|
|
assert_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
|
|
remove_file 'lib/mailer_previews/notifier_preview.rb'
|
|
sleep(1)
|
|
|
|
get "/rails/mailers"
|
|
assert_no_match '<h3><a href="/rails/mailers/notifier">Notifier</a></h3>', last_response.body
|
|
end
|
|
|
|
test "mailer preview not found" do
|
|
app('development')
|
|
get "/rails/mailers/notifier"
|
|
assert last_response.not_found?
|
|
assert_match "Mailer preview 'notifier' not found", last_response.body
|
|
end
|
|
|
|
test "mailer preview email not found" do
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers/notifier/bar"
|
|
assert last_response.not_found?
|
|
assert_match "Email 'bar' not found in NotifierPreview", last_response.body
|
|
end
|
|
|
|
test "mailer preview email part not found" do
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers/notifier/foo?part=text%2Fhtml"
|
|
assert last_response.not_found?
|
|
assert_match "Email part 'text/html' not found in NotifierPreview#foo", last_response.body
|
|
end
|
|
|
|
test "message header uses full display names" do
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "Ruby on Rails <core@rubyonrails.org>"
|
|
|
|
def foo
|
|
mail to: "Andrew White <andyw@pixeltrix.co.uk>",
|
|
cc: "David Heinemeier Hansson <david@heinemeierhansson.com>"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers/notifier/foo"
|
|
assert_equal 200, last_response.status
|
|
assert_match "Ruby on Rails <core@rubyonrails.org>", last_response.body
|
|
assert_match "Andrew White <andyw@pixeltrix.co.uk>", last_response.body
|
|
assert_match "David Heinemeier Hansson <david@heinemeierhansson.com>", last_response.body
|
|
end
|
|
|
|
test "part menu selects correct option" do
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def foo
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
html_template 'notifier/foo', <<-RUBY
|
|
<p>Hello, World!</p>
|
|
RUBY
|
|
|
|
text_template 'notifier/foo', <<-RUBY
|
|
Hello, World!
|
|
RUBY
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def foo
|
|
Notifier.foo
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
get "/rails/mailers/notifier/foo.html"
|
|
assert_equal 200, last_response.status
|
|
assert_match '<option selected value="?part=text%2Fhtml">View as HTML email</option>', last_response.body
|
|
|
|
get "/rails/mailers/notifier/foo.txt"
|
|
assert_equal 200, last_response.status
|
|
assert_match '<option selected value="?part=text%2Fplain">View as plain-text email</option>', last_response.body
|
|
end
|
|
|
|
test "*_path helpers emit a deprecation" do
|
|
|
|
app_file "config/routes.rb", <<-RUBY
|
|
Rails.application.routes.draw do
|
|
get 'foo', to: 'foo#index'
|
|
end
|
|
RUBY
|
|
|
|
mailer 'notifier', <<-RUBY
|
|
class Notifier < ActionMailer::Base
|
|
default from: "from@example.com"
|
|
|
|
def path_in_view
|
|
mail to: "to@example.org"
|
|
end
|
|
|
|
def path_in_mailer
|
|
@url = foo_path
|
|
mail to: "to@example.org"
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
html_template 'notifier/path_in_view', "<%= link_to 'foo', foo_path %>"
|
|
|
|
mailer_preview 'notifier', <<-RUBY
|
|
class NotifierPreview < ActionMailer::Preview
|
|
def path_in_view
|
|
Notifier.path_in_view
|
|
end
|
|
|
|
def path_in_mailer
|
|
Notifier.path_in_mailer
|
|
end
|
|
end
|
|
RUBY
|
|
|
|
app('development')
|
|
|
|
assert_deprecated do
|
|
get "/rails/mailers/notifier/path_in_view.html"
|
|
assert_equal 200, last_response.status
|
|
end
|
|
|
|
html_template 'notifier/path_in_mailer', "No ERB in here"
|
|
|
|
assert_deprecated do
|
|
get "/rails/mailers/notifier/path_in_mailer.html"
|
|
assert_equal 200, last_response.status
|
|
end
|
|
end
|
|
|
|
private
|
|
def build_app
|
|
super
|
|
app_file "config/routes.rb", "Rails.application.routes.draw do; end"
|
|
end
|
|
|
|
def mailer(name, contents)
|
|
app_file("app/mailers/#{name}.rb", contents)
|
|
end
|
|
|
|
def mailer_preview(name, contents)
|
|
app_file("test/mailers/previews/#{name}_preview.rb", contents)
|
|
end
|
|
|
|
def html_template(name, contents)
|
|
app_file("app/views/#{name}.html.erb", contents)
|
|
end
|
|
|
|
def text_template(name, contents)
|
|
app_file("app/views/#{name}.text.erb", contents)
|
|
end
|
|
end
|
|
end
|