mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
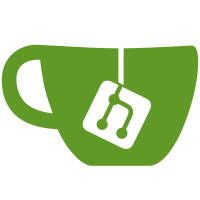
We sometimes say "✂️ newline after `private`" in a code review (e.g. https://github.com/rails/rails/pull/18546#discussion_r23188776, https://github.com/rails/rails/pull/34832#discussion_r244847195). Now `Layout/EmptyLinesAroundAccessModifier` cop have new enforced style `EnforcedStyle: only_before` (https://github.com/rubocop-hq/rubocop/pull/7059). That cop and enforced style will reduce the our code review cost.
74 lines
1.9 KiB
Ruby
74 lines
1.9 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "active_support/callbacks"
|
|
require "active_support/core_ext/module/attribute_accessors_per_thread"
|
|
require "concurrent"
|
|
|
|
module ActionCable
|
|
module Server
|
|
# Worker used by Server.send_async to do connection work in threads.
|
|
class Worker # :nodoc:
|
|
include ActiveSupport::Callbacks
|
|
|
|
thread_mattr_accessor :connection
|
|
define_callbacks :work
|
|
include ActiveRecordConnectionManagement
|
|
|
|
attr_reader :executor
|
|
|
|
def initialize(max_size: 5)
|
|
@executor = Concurrent::ThreadPoolExecutor.new(
|
|
min_threads: 1,
|
|
max_threads: max_size,
|
|
max_queue: 0,
|
|
)
|
|
end
|
|
|
|
# Stop processing work: any work that has not already started
|
|
# running will be discarded from the queue
|
|
def halt
|
|
@executor.shutdown
|
|
end
|
|
|
|
def stopping?
|
|
@executor.shuttingdown?
|
|
end
|
|
|
|
def work(connection)
|
|
self.connection = connection
|
|
|
|
run_callbacks :work do
|
|
yield
|
|
end
|
|
ensure
|
|
self.connection = nil
|
|
end
|
|
|
|
def async_exec(receiver, *args, connection:, &block)
|
|
async_invoke receiver, :instance_exec, *args, connection: connection, &block
|
|
end
|
|
|
|
def async_invoke(receiver, method, *args, connection: receiver, &block)
|
|
@executor.post do
|
|
invoke(receiver, method, *args, connection: connection, &block)
|
|
end
|
|
end
|
|
|
|
def invoke(receiver, method, *args, connection:, &block)
|
|
work(connection) do
|
|
receiver.send method, *args, &block
|
|
rescue Exception => e
|
|
logger.error "There was an exception - #{e.class}(#{e.message})"
|
|
logger.error e.backtrace.join("\n")
|
|
|
|
receiver.handle_exception if receiver.respond_to?(:handle_exception)
|
|
end
|
|
end
|
|
|
|
private
|
|
def logger
|
|
ActionCable.server.logger
|
|
end
|
|
end
|
|
end
|
|
end
|