mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
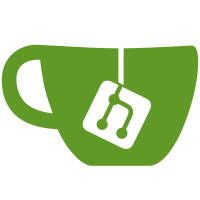
This commit renames `RoleManager` -> `PoolManager` and `Role` -> `PoolConfig`. Once we introduced the previous commit, and looking at the existing code, it's clearer that `Role` and `RoleManager` are not the right names for these. Since this PR moves away from swapping the connection handler concepts around and the role concept will continue existing on the handler level, we need to rename this. A `PoolConfig` holds a `connection_specification_name` (we may rename this down the road), a `db_config`, a `schema_cache`, and a `pool`. It does feel like `pool` could eventually hold all of these things instead of having a `PoolConfig` object. This would remove one level of the object graph and reduce complexity. For now I'm leaving this object to keep the change churn low and will revisit later. Co-authored-by: John Crepezzi <seejohnrun@github.com>
46 lines
1.2 KiB
Ruby
46 lines
1.2 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "cases/helper"
|
|
|
|
class TestRecord < ActiveRecord::Base
|
|
end
|
|
|
|
class TestUnconnectedAdapter < ActiveRecord::TestCase
|
|
self.use_transactional_tests = false
|
|
|
|
def setup
|
|
@underlying = ActiveRecord::Base.connection
|
|
@specification = ActiveRecord::Base.remove_connection
|
|
|
|
# Clear out connection info from other pids (like a fork parent) too
|
|
ActiveRecord::ConnectionAdapters::PoolConfig.discard_pools!
|
|
end
|
|
|
|
teardown do
|
|
@underlying = nil
|
|
ActiveRecord::Base.establish_connection(@specification)
|
|
load_schema if in_memory_db?
|
|
end
|
|
|
|
def test_connection_no_longer_established
|
|
assert_raise(ActiveRecord::ConnectionNotEstablished) do
|
|
TestRecord.find(1)
|
|
end
|
|
|
|
assert_raise(ActiveRecord::ConnectionNotEstablished) do
|
|
TestRecord.new.save
|
|
end
|
|
end
|
|
|
|
def test_error_message_when_connection_not_established
|
|
error = assert_raise(ActiveRecord::ConnectionNotEstablished) do
|
|
TestRecord.find(1)
|
|
end
|
|
|
|
assert_equal "No connection pool with 'primary' found.", error.message
|
|
end
|
|
|
|
def test_underlying_adapter_no_longer_active
|
|
assert_not @underlying.active?, "Removed adapter should no longer be active"
|
|
end
|
|
end
|