mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
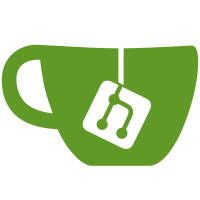
If a preview cannot be generated, the IO stream that is captured is empty, resulting in a 0-byte preview file being generated and stored in the Active Storage service. We came across this because Poppler was failing to generate previews of some PDFs, resulting in 0-byte files. Resizing those "previews" then resulted in a MiniMagick error. The MiniMagick error feels like the right end result if it's attempted on a 0-byte file, what doesn't feel right is `Previewer` proceeding normally if the child process that attempted to capture a preview exited unsuccessfully. Now, if the previewer child process exits with a non-0 status code, we raise an exception.
42 lines
1.4 KiB
Ruby
42 lines
1.4 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "test_helper"
|
|
require "database/setup"
|
|
|
|
require "active_storage/previewer/poppler_pdf_previewer"
|
|
|
|
class ActiveStorage::Previewer::PopplerPDFPreviewerTest < ActiveSupport::TestCase
|
|
test "previewing a PDF document" do
|
|
blob = create_file_blob(filename: "report.pdf", content_type: "application/pdf")
|
|
|
|
ActiveStorage::Previewer::PopplerPDFPreviewer.new(blob).preview do |attachable|
|
|
assert_equal "image/png", attachable[:content_type]
|
|
assert_equal "report.png", attachable[:filename]
|
|
|
|
image = MiniMagick::Image.read(attachable[:io])
|
|
assert_equal 612, image.width
|
|
assert_equal 792, image.height
|
|
end
|
|
end
|
|
|
|
test "previewing a cropped PDF document" do
|
|
blob = create_file_blob(filename: "cropped.pdf", content_type: "application/pdf")
|
|
|
|
ActiveStorage::Previewer::PopplerPDFPreviewer.new(blob).preview do |attachable|
|
|
assert_equal "image/png", attachable[:content_type]
|
|
assert_equal "cropped.png", attachable[:filename]
|
|
|
|
image = MiniMagick::Image.read(attachable[:io])
|
|
assert_equal 430, image.width
|
|
assert_equal 145, image.height
|
|
end
|
|
end
|
|
|
|
test "previewing a PDF that can't be previewed" do
|
|
blob = create_file_blob(filename: "video.mp4", content_type: "application/pdf")
|
|
|
|
assert_raises ActiveStorage::PreviewError do
|
|
ActiveStorage::Previewer::PopplerPDFPreviewer.new(blob).preview
|
|
end
|
|
end
|
|
end
|