mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
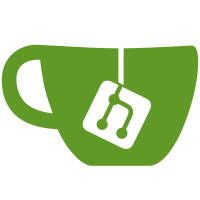
I ran into an issue where validations on a suppressed record were causing validation errors to be thrown on a record that was never going to be saved. There isn't a reason to run the validations on a record that doesn't matter. This change moves the suppressor up the chain to be run on the `save` or `save!` in the validations rather than in persistence. The issue with running it when we hit persistence is that the validations are run first, then we hit persistance, and then we hit the suppressor. The suppressor comes first. The change to the test was required since I added the `validates_presence_of` validations. Adding this alone was enough to demonstrate the issue. I added a new test to demonstrate the new behavior is explict.
63 lines
1.5 KiB
Ruby
63 lines
1.5 KiB
Ruby
require 'cases/helper'
|
|
require 'models/notification'
|
|
require 'models/user'
|
|
|
|
class SuppressorTest < ActiveRecord::TestCase
|
|
def test_suppresses_create
|
|
assert_no_difference -> { Notification.count } do
|
|
Notification.suppress do
|
|
Notification.create
|
|
Notification.create!
|
|
Notification.new.save
|
|
Notification.new.save!
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_suppresses_update
|
|
user = User.create! token: 'asdf'
|
|
|
|
User.suppress do
|
|
user.update token: 'ghjkl'
|
|
assert_equal 'asdf', user.reload.token
|
|
|
|
user.update! token: 'zxcvbnm'
|
|
assert_equal 'asdf', user.reload.token
|
|
|
|
user.token = 'qwerty'
|
|
user.save
|
|
assert_equal 'asdf', user.reload.token
|
|
|
|
user.token = 'uiop'
|
|
user.save!
|
|
assert_equal 'asdf', user.reload.token
|
|
end
|
|
end
|
|
|
|
def test_suppresses_create_in_callback
|
|
assert_difference -> { User.count } do
|
|
assert_no_difference -> { Notification.count } do
|
|
Notification.suppress { UserWithNotification.create! }
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_resumes_saving_after_suppression_complete
|
|
Notification.suppress { UserWithNotification.create! }
|
|
|
|
assert_difference -> { Notification.count } do
|
|
Notification.create!(message: "New Comment")
|
|
end
|
|
end
|
|
|
|
def test_suppresses_validations_on_create
|
|
assert_no_difference -> { Notification.count } do
|
|
Notification.suppress do
|
|
User.create
|
|
User.create!
|
|
User.new.save
|
|
User.new.save!
|
|
end
|
|
end
|
|
end
|
|
end
|