mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
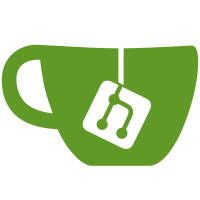
This was caused by 6d0d83a33f
. While the
bug it's trying to fix is handled if the association is loaded in an
after_(create|save) callback, it doesn't handle any cases that load the
association before the persistence takes place (validation, or before_*
filters). Instead of caring about the timing of persistence, we can just
ensure that we're not double adding the record instead.
The test from that commit actually broke, but it was not because the bug
has been re-introduced. It was because `Bulb` in our test suite is doing
funky things that look like STI but isn't STI, so equality comparison
didn't happen as the loaded model was of a different class.
Fixes #26661.
52 lines
1.1 KiB
Ruby
52 lines
1.1 KiB
Ruby
class Bulb < ActiveRecord::Base
|
|
default_scope { where(name: "defaulty") }
|
|
belongs_to :car, touch: true
|
|
scope :awesome, -> { where(frickinawesome: true) }
|
|
|
|
attr_reader :scope_after_initialize, :attributes_after_initialize
|
|
|
|
after_initialize :record_scope_after_initialize
|
|
def record_scope_after_initialize
|
|
@scope_after_initialize = self.class.all
|
|
end
|
|
|
|
after_initialize :record_attributes_after_initialize
|
|
def record_attributes_after_initialize
|
|
@attributes_after_initialize = attributes.dup
|
|
end
|
|
|
|
def color=(color)
|
|
self[:color] = color.upcase + "!"
|
|
end
|
|
|
|
def self.new(attributes = {}, &block)
|
|
bulb_type = (attributes || {}).delete(:bulb_type)
|
|
|
|
if bulb_type.present?
|
|
bulb_class = "#{bulb_type.to_s.camelize}Bulb".constantize
|
|
bulb_class.new(attributes, &block)
|
|
else
|
|
super
|
|
end
|
|
end
|
|
end
|
|
|
|
class CustomBulb < Bulb
|
|
after_initialize :set_awesomeness
|
|
|
|
def set_awesomeness
|
|
self.frickinawesome = true if name == "Dude"
|
|
end
|
|
end
|
|
|
|
class FunkyBulb < Bulb
|
|
before_destroy do
|
|
raise "before_destroy was called"
|
|
end
|
|
end
|
|
|
|
class FailedBulb < Bulb
|
|
before_destroy do
|
|
throw(:abort)
|
|
end
|
|
end
|