mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
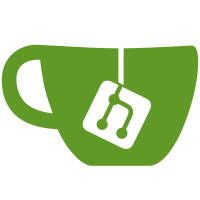
Previously, freezing a cloned ActiveRecord object froze the original too. By cloning `@attributes` before freezing, we prevent cloned objects (which in Ruby share state of ivars) from being effected by `#freeze`. Resolves issue #4936, which has further information on this issue, as well as steps to reproduce. * Add a test case for `#freeze` not causing `cloned.frozen?` to be true. * Clone @attributes before freezing in `ActiveRecord::Core`, then reassign the cloned, frozen hash to the frozen model's `@attributes` ivar. /cc @steveklabnik
40 lines
978 B
Ruby
40 lines
978 B
Ruby
require "cases/helper"
|
|
require 'models/topic'
|
|
|
|
module ActiveRecord
|
|
class CloneTest < ActiveRecord::TestCase
|
|
fixtures :topics
|
|
|
|
def test_persisted
|
|
topic = Topic.first
|
|
cloned = topic.clone
|
|
assert topic.persisted?, 'topic persisted'
|
|
assert cloned.persisted?, 'topic persisted'
|
|
assert !cloned.new_record?, 'topic is not new'
|
|
end
|
|
|
|
def test_stays_frozen
|
|
topic = Topic.first
|
|
topic.freeze
|
|
|
|
cloned = topic.clone
|
|
assert cloned.persisted?, 'topic persisted'
|
|
assert !cloned.new_record?, 'topic is not new'
|
|
assert cloned.frozen?, 'topic should be frozen'
|
|
end
|
|
|
|
def test_shallow
|
|
topic = Topic.first
|
|
cloned = topic.clone
|
|
topic.author_name = 'Aaron'
|
|
assert_equal 'Aaron', cloned.author_name
|
|
end
|
|
|
|
def test_freezing_a_cloned_model_does_not_freeze_clone
|
|
cloned = Topic.new
|
|
clone = cloned.clone
|
|
cloned.freeze
|
|
assert_not clone.frozen?
|
|
end
|
|
end
|
|
end
|