mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
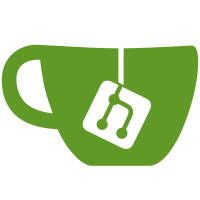
Before this commit, only StandardError exceptions can be handled by rescue_from handlers. This changes the rescue clause to catch all Exception objects, allowing rescue handlers to be defined for Exception classes not inheriting from StandardError. This means that rescue handlers that are rescuing Exceptions outside of StandardError exceptions may rescue exceptions that were not being rescued before this change. Co-authored-by: Adrianna Chang <adrianna.chang@shopify.com>
41 lines
1.3 KiB
Ruby
41 lines
1.3 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "helper"
|
|
require "jobs/rescue_job"
|
|
require "models/person"
|
|
|
|
class RescueTest < ActiveSupport::TestCase
|
|
setup do
|
|
JobBuffer.clear
|
|
end
|
|
|
|
test "rescue perform exception with retry" do
|
|
job = RescueJob.new("david")
|
|
job.perform_now
|
|
assert_equal [ "rescued from ArgumentError", "performed beautifully", "Retried job DIFFERENT!" ], JobBuffer.values
|
|
end
|
|
|
|
test "let through unhandled perform exception" do
|
|
job = RescueJob.new("other")
|
|
assert_raises(RescueJob::OtherError) do
|
|
job.perform_now
|
|
end
|
|
end
|
|
|
|
test "rescue from deserialization errors" do
|
|
RescueJob.perform_later Person.new(404)
|
|
assert_includes JobBuffer.values, "rescued from DeserializationError"
|
|
assert_includes JobBuffer.values, "DeserializationError original exception was Person::RecordNotFound"
|
|
assert_not_includes JobBuffer.values, "performed beautifully"
|
|
end
|
|
|
|
test "should not wrap DeserializationError in DeserializationError" do
|
|
RescueJob.perform_later [Person.new(404)]
|
|
assert_includes JobBuffer.values, "DeserializationError original exception was Person::RecordNotFound"
|
|
end
|
|
|
|
test "rescue from exceptions that don't inherit from StandardError" do
|
|
RescueJob.perform_later("rafael")
|
|
assert_equal ["rescued from NotImplementedError"], JobBuffer.values
|
|
end
|
|
end
|