mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
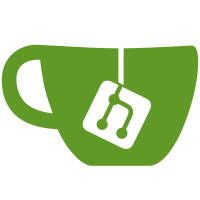
I’m renaming all instances of `use_transcational_fixtures` to `use_transactional_tests` and “transactional fixtures” to “transactional tests”. I’m deprecating `use_transactional_fixtures=`. So anyone who is explicitly setting this will get a warning telling them to use `use_transactional_tests=` instead. I’m maintaining backwards compatibility—both forms will work. `use_transactional_tests` will check to see if `use_transactional_fixtures` is set and use that, otherwise it will use itself. But because `use_transactional_tests` is a class attribute (created with `class_attribute`) this requires a little bit of hoop jumping. The writer method that `class_attribute` generates defines a new reader method that return the value being set. Which means we can’t set the default of `true` using `use_transactional_tests=` as was done previously because that won’t take into account anyone using `use_transactional_fixtures`. Instead I defined the reader method manually and it checks `use_transactional_fixtures`. If it was set then it should be used, otherwise it should return the default, which is `true`. If someone uses `use_transactional_tests=` then it will overwrite the backwards-compatible method with whatever they set.
102 lines
2.7 KiB
Ruby
102 lines
2.7 KiB
Ruby
require "cases/helper"
|
|
|
|
class RequiredAssociationsTest < ActiveRecord::TestCase
|
|
self.use_transactional_tests = false
|
|
|
|
class Parent < ActiveRecord::Base
|
|
end
|
|
|
|
class Child < ActiveRecord::Base
|
|
end
|
|
|
|
setup do
|
|
@connection = ActiveRecord::Base.connection
|
|
@connection.create_table :parents, force: true
|
|
@connection.create_table :children, force: true do |t|
|
|
t.belongs_to :parent
|
|
end
|
|
end
|
|
|
|
teardown do
|
|
@connection.drop_table 'parents', if_exists: true
|
|
@connection.drop_table 'children', if_exists: true
|
|
end
|
|
|
|
test "belongs_to associations are not required by default" do
|
|
model = subclass_of(Child) do
|
|
belongs_to :parent, inverse_of: false,
|
|
class_name: "RequiredAssociationsTest::Parent"
|
|
end
|
|
|
|
assert model.new.save
|
|
assert model.new(parent: Parent.new).save
|
|
end
|
|
|
|
test "required belongs_to associations have presence validated" do
|
|
model = subclass_of(Child) do
|
|
belongs_to :parent, required: true, inverse_of: false,
|
|
class_name: "RequiredAssociationsTest::Parent"
|
|
end
|
|
|
|
record = model.new
|
|
assert_not record.save
|
|
assert_equal ["Parent must exist"], record.errors.full_messages
|
|
|
|
record.parent = Parent.new
|
|
assert record.save
|
|
end
|
|
|
|
test "has_one associations are not required by default" do
|
|
model = subclass_of(Parent) do
|
|
has_one :child, inverse_of: false,
|
|
class_name: "RequiredAssociationsTest::Child"
|
|
end
|
|
|
|
assert model.new.save
|
|
assert model.new(child: Child.new).save
|
|
end
|
|
|
|
test "required has_one associations have presence validated" do
|
|
model = subclass_of(Parent) do
|
|
has_one :child, required: true, inverse_of: false,
|
|
class_name: "RequiredAssociationsTest::Child"
|
|
end
|
|
|
|
record = model.new
|
|
assert_not record.save
|
|
assert_equal ["Child must exist"], record.errors.full_messages
|
|
|
|
record.child = Child.new
|
|
assert record.save
|
|
end
|
|
|
|
test "required has_one associations have a correct error message" do
|
|
model = subclass_of(Parent) do
|
|
has_one :child, required: true, inverse_of: false,
|
|
class_name: "RequiredAssociationsTest::Child"
|
|
end
|
|
|
|
record = model.create
|
|
assert_equal ["Child must exist"], record.errors.full_messages
|
|
end
|
|
|
|
test "required belongs_to associations have a correct error message" do
|
|
model = subclass_of(Child) do
|
|
belongs_to :parent, required: true, inverse_of: false,
|
|
class_name: "RequiredAssociationsTest::Parent"
|
|
end
|
|
|
|
record = model.create
|
|
assert_equal ["Parent must exist"], record.errors.full_messages
|
|
end
|
|
|
|
private
|
|
|
|
def subclass_of(klass, &block)
|
|
subclass = Class.new(klass, &block)
|
|
def subclass.name
|
|
superclass.name
|
|
end
|
|
subclass
|
|
end
|
|
end
|