mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
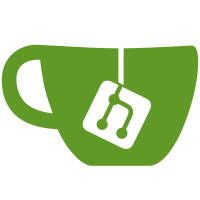
Selecting which key extensions to include in active_support/rails
made apparent the systematic usage of Object#in? in the code base.
After some discussion in
5ea6b0df9a
we decided to remove it and use plain Ruby, which seems enough
for this particular idiom.
In this commit the refactor has been made case by case. Sometimes
include? is the natural alternative, others a simple || is the
way you actually spell the condition in your head, others a case
statement seems more appropriate. I have chosen the one I liked
the most in each case.
60 lines
1.6 KiB
Ruby
60 lines
1.6 KiB
Ruby
require 'abstract_unit'
|
|
|
|
class ErbUtilTest < ActiveSupport::TestCase
|
|
include ERB::Util
|
|
|
|
ERB::Util::HTML_ESCAPE.each do |given, expected|
|
|
define_method "test_html_escape_#{expected.gsub(/\W/, '')}" do
|
|
assert_equal expected, html_escape(given)
|
|
end
|
|
end
|
|
|
|
ERB::Util::JSON_ESCAPE.each do |given, expected|
|
|
define_method "test_json_escape_#{expected.gsub(/\W/, '')}" do
|
|
assert_equal ERB::Util::JSON_ESCAPE[given], json_escape(given)
|
|
end
|
|
end
|
|
|
|
def test_json_escape_returns_unsafe_strings_when_passed_unsafe_strings
|
|
value = json_escape("asdf")
|
|
assert !value.html_safe?
|
|
end
|
|
|
|
def test_json_escape_returns_safe_strings_when_passed_safe_strings
|
|
value = json_escape("asdf".html_safe)
|
|
assert value.html_safe?
|
|
end
|
|
|
|
def test_html_escape_is_html_safe
|
|
escaped = h("<p>")
|
|
assert_equal "<p>", escaped
|
|
assert escaped.html_safe?
|
|
end
|
|
|
|
def test_html_escape_passes_html_escpe_unmodified
|
|
escaped = h("<p>".html_safe)
|
|
assert_equal "<p>", escaped
|
|
assert escaped.html_safe?
|
|
end
|
|
|
|
def test_rest_in_ascii
|
|
(0..127).to_a.map {|int| int.chr }.each do |chr|
|
|
next if %('"&<>).include?(chr)
|
|
assert_equal chr, html_escape(chr)
|
|
end
|
|
end
|
|
|
|
def test_html_escape_once
|
|
assert_equal '1 <>&"' 2 & 3', html_escape_once('1 <>&"\' 2 & 3')
|
|
end
|
|
|
|
def test_html_escape_once_returns_unsafe_strings_when_passed_unsafe_strings
|
|
value = html_escape_once('1 < 2 & 3')
|
|
assert !value.html_safe?
|
|
end
|
|
|
|
def test_html_escape_once_returns_safe_strings_when_passed_safe_strings
|
|
value = html_escape_once('1 < 2 & 3'.html_safe)
|
|
assert value.html_safe?
|
|
end
|
|
end
|