mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
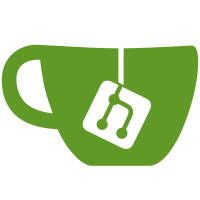
- ### Problem ```ruby MyJob < ApplicationJob before_enqueue { throw(:abort) } after_enqueue { # enters here } end ``` I find AJ behaviour on after_enqueue and after_perform callbacks weird as they get run even when the callback chain is halted. It's counter intuitive to run the after_enqueue callbacks even though the job wasn't event enqueued. ### Solution In Rails 6.2, I propose to make the new behaviour the default and stop running after callbacks when the chain is halted. For application that wants this behaviour now or in 6.1 they can do so by adding the `config.active_job.skip_after_callbacks_if_terminated = true` in their configuration file.
24 lines
498 B
Ruby
24 lines
498 B
Ruby
# frozen_string_literal: true
|
|
|
|
class AbortBeforeEnqueueJob < ActiveJob::Base
|
|
MyError = Class.new(StandardError)
|
|
|
|
before_enqueue :throw_or_raise
|
|
after_enqueue { self.flag = "after_enqueue" }
|
|
before_perform { throw(:abort) }
|
|
after_perform { self.flag = "after_perform" }
|
|
|
|
attr_accessor :flag
|
|
|
|
def perform
|
|
raise "This should never be called"
|
|
end
|
|
|
|
def throw_or_raise
|
|
if (arguments.first || :abort) == :abort
|
|
throw(:abort)
|
|
else
|
|
raise(MyError)
|
|
end
|
|
end
|
|
end
|