mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
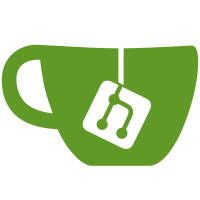
I have so. many. regrets. about using `spec_name` for database configurations and now I'm finally putting this mistake to an end. Back when I started multi-db work I assumed that eventually `connection_specification_name` (sometimes called `spec_name`) and `spec_name` for configurations would one day be the same thing. After 2 years I no longer believe they will ever be the same thing. This PR deprecates `spec_name` on database configurations in favor of `name`. It's the same behavior, just a better name, or at least a less confusing name. `connection_specification_name` refers to the parent class name (ie ActiveRecord::Base, AnimalsBase, etc) that holds the connection for it's models. In some places like ConnectionHandler it shortens this to `spec_name`, hence the major confusion. Recently I've been working with some new folks on database stuff and connection management and realize how confusing it was to explain that `db_config.spec_name` was not `spec_name` and `connection_specification_name`. Worse than that one is a symbole while the other is a class name. This was made even more complicated by the fact that `ActiveRecord::Base` used `primary` as the `connection_specification_name` until #38190. After spending 2 years with connection management I don't believe that we can ever use the symbols from the database configs as a way to connect the database without the class name being _somewhere_ because a db_config does not know who it's owner class is until it's been connected and a model has no idea what db_config belongs to it until it's connected. The model is the only way to tie a primary/writer config to a replica/reader config. This could change in the future but I don't see value in adding a class name to the db_configs before connection or telling a model what config belongs to it before connection. That would probably break a lot of application assumptions. If we do ever end up in that world, we can use name, because tbh `spec_name` and `connection_specification_name` were always confusing to me.
90 lines
2.9 KiB
Ruby
90 lines
2.9 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "isolation/abstract_unit"
|
|
|
|
module ApplicationTests
|
|
class HooksTest < ActiveSupport::TestCase
|
|
include ActiveSupport::Testing::Isolation
|
|
|
|
def setup
|
|
build_app
|
|
FileUtils.rm_rf "#{app_path}/config/environments"
|
|
end
|
|
|
|
def teardown
|
|
teardown_app
|
|
end
|
|
|
|
test "load initializers" do
|
|
app_file "config/initializers/foo.rb", "$foo = true"
|
|
require "#{app_path}/config/environment"
|
|
assert $foo
|
|
end
|
|
|
|
test "hooks block works correctly without eager_load (before_eager_load is not called)" do
|
|
add_to_config <<-RUBY
|
|
$initialization_callbacks = []
|
|
config.root = "#{app_path}"
|
|
config.eager_load = false
|
|
config.before_configuration { $initialization_callbacks << 1 }
|
|
config.before_initialize { $initialization_callbacks << 2 }
|
|
config.before_eager_load { Boom }
|
|
config.after_initialize { $initialization_callbacks << 3 }
|
|
RUBY
|
|
|
|
require "#{app_path}/config/environment"
|
|
assert_equal [1, 2, 3], $initialization_callbacks
|
|
end
|
|
|
|
test "hooks block works correctly with eager_load" do
|
|
add_to_config <<-RUBY
|
|
$initialization_callbacks = []
|
|
config.root = "#{app_path}"
|
|
config.eager_load = true
|
|
config.before_configuration { $initialization_callbacks << 1 }
|
|
config.before_initialize { $initialization_callbacks << 2 }
|
|
config.before_eager_load { $initialization_callbacks << 3 }
|
|
config.after_initialize { $initialization_callbacks << 4 }
|
|
RUBY
|
|
|
|
require "#{app_path}/config/environment"
|
|
assert_equal [1, 2, 3, 4], $initialization_callbacks
|
|
end
|
|
|
|
test "after_initialize runs after frameworks have been initialized" do
|
|
$activerecord_configuration = nil
|
|
add_to_config <<-RUBY
|
|
config.after_initialize { $activerecord_configuration = ActiveRecord::Base.configurations.configs_for(env_name: "development", name: "primary") }
|
|
RUBY
|
|
|
|
require "#{app_path}/config/environment"
|
|
assert $activerecord_configuration
|
|
end
|
|
|
|
test "after_initialize happens after to_prepare in development" do
|
|
$order = []
|
|
add_to_config <<-RUBY
|
|
config.cache_classes = false
|
|
config.after_initialize { $order << :after_initialize }
|
|
config.to_prepare { $order << :to_prepare }
|
|
RUBY
|
|
|
|
require "#{app_path}/config/environment"
|
|
assert_equal [:to_prepare, :after_initialize], $order
|
|
end
|
|
|
|
test "after_initialize happens after to_prepare in production" do
|
|
$order = []
|
|
add_to_config <<-RUBY
|
|
config.cache_classes = true
|
|
config.after_initialize { $order << :after_initialize }
|
|
config.to_prepare { $order << :to_prepare }
|
|
RUBY
|
|
|
|
require "#{app_path}/config/application"
|
|
Rails.env.replace "production"
|
|
require "#{app_path}/config/environment"
|
|
assert_equal [:to_prepare, :after_initialize], $order
|
|
end
|
|
end
|
|
end
|