mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
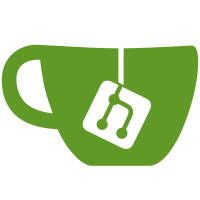
The native timestamp type in MySQL is different from datetime type. Internal representation of the timestamp type is UNIX time, This means that timestamp columns are affected by time zone. ``` > SET time_zone = '+00:00'; Query OK, 0 rows affected (0.00 sec) > INSERT INTO time_with_zone(ts,dt) VALUES (NOW(),NOW()); Query OK, 1 row affected (0.02 sec) > SELECT * FROM time_with_zone; +---------------------+---------------------+ | ts | dt | +---------------------+---------------------+ | 2016-02-07 22:11:44 | 2016-02-07 22:11:44 | +---------------------+---------------------+ 1 row in set (0.00 sec) > SET time_zone = '-08:00'; Query OK, 0 rows affected (0.00 sec) > SELECT * FROM time_with_zone; +---------------------+---------------------+ | ts | dt | +---------------------+---------------------+ | 2016-02-07 14:11:44 | 2016-02-07 22:11:44 | +---------------------+---------------------+ 1 row in set (0.00 sec) ```
73 lines
2 KiB
Ruby
73 lines
2 KiB
Ruby
ActiveRecord::Schema.define do
|
|
|
|
if ActiveRecord::Base.connection.version >= "5.6.0"
|
|
create_table :datetime_defaults, force: true do |t|
|
|
t.datetime :modified_datetime, default: -> { "CURRENT_TIMESTAMP" }
|
|
end
|
|
end
|
|
|
|
create_table :timestamp_defaults, force: true do |t|
|
|
t.timestamp :nullable_timestamp
|
|
t.timestamp :modified_timestamp, default: -> { "CURRENT_TIMESTAMP" }
|
|
end
|
|
|
|
create_table :binary_fields, force: true do |t|
|
|
t.binary :var_binary, limit: 255
|
|
t.binary :var_binary_large, limit: 4095
|
|
t.tinyblob :tiny_blob
|
|
t.blob :normal_blob
|
|
t.mediumblob :medium_blob
|
|
t.longblob :long_blob
|
|
t.tinytext :tiny_text
|
|
t.text :normal_text
|
|
t.mediumtext :medium_text
|
|
t.longtext :long_text
|
|
|
|
t.index :var_binary
|
|
end
|
|
|
|
create_table :key_tests, force: true, options: "ENGINE=MyISAM" do |t|
|
|
t.string :awesome
|
|
t.string :pizza
|
|
t.string :snacks
|
|
t.index :awesome, type: :fulltext, name: "index_key_tests_on_awesome"
|
|
t.index :pizza, using: :btree, name: "index_key_tests_on_pizza"
|
|
t.index :snacks, name: "index_key_tests_on_snack"
|
|
end
|
|
|
|
create_table :collation_tests, id: false, force: true do |t|
|
|
t.string :string_cs_column, limit: 1, collation: "utf8_bin"
|
|
t.string :string_ci_column, limit: 1, collation: "utf8_general_ci"
|
|
t.binary :binary_column, limit: 1
|
|
end
|
|
|
|
ActiveRecord::Base.connection.execute <<-SQL
|
|
DROP PROCEDURE IF EXISTS ten;
|
|
SQL
|
|
|
|
ActiveRecord::Base.connection.execute <<-SQL
|
|
CREATE PROCEDURE ten() SQL SECURITY INVOKER
|
|
BEGIN
|
|
select 10;
|
|
END
|
|
SQL
|
|
|
|
ActiveRecord::Base.connection.execute <<-SQL
|
|
DROP PROCEDURE IF EXISTS topics;
|
|
SQL
|
|
|
|
ActiveRecord::Base.connection.execute <<-SQL
|
|
CREATE PROCEDURE topics(IN num INT) SQL SECURITY INVOKER
|
|
BEGIN
|
|
select * from topics limit num;
|
|
END
|
|
SQL
|
|
|
|
ActiveRecord::Base.connection.drop_table "enum_tests", if_exists: true
|
|
|
|
ActiveRecord::Base.connection.execute <<-SQL
|
|
CREATE TABLE enum_tests (
|
|
enum_column ENUM('text','blob','tiny','medium','long','unsigned','bigint')
|
|
)
|
|
SQL
|
|
end
|