mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
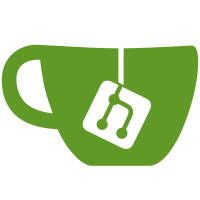
* In Rails 2.3 apps being upgraded, you will need to add the deprecation configuration to each of your environments. Failing to do so will result in the same behavior as Rails 2.3, but with an outputted warning to provide information on how to set up the setting. * New Rails 3 applications generate the setting * The notification style will send deprecation notices using ActiveSupport::Notifications. Third-party tools can listen in to these notifications to provide a streamlined view of the deprecation notices occurring in your app. * The payload in the notification is the deprecation warning itself as well as the callstack from the point that triggered the notification.
58 lines
No EOL
2.1 KiB
Ruby
58 lines
No EOL
2.1 KiB
Ruby
require "active_support"
|
|
require "rails"
|
|
require "active_support/i18n_railtie"
|
|
|
|
module ActiveSupport
|
|
class Railtie < Rails::Railtie
|
|
config.active_support = ActiveSupport::OrderedOptions.new
|
|
|
|
# Loads support for "whiny nil" (noisy warnings when methods are invoked
|
|
# on +nil+ values) if Configuration#whiny_nils is true.
|
|
initializer "active_support.initialize_whiny_nils" do |app|
|
|
require 'active_support/whiny_nil' if app.config.whiny_nils
|
|
end
|
|
|
|
initializer "active_support.deprecation_behavior" do |app|
|
|
if deprecation = app.config.active_support.deprecation
|
|
ActiveSupport::Deprecation.behavior = deprecation
|
|
else
|
|
defaults = {"development" => :log,
|
|
"production" => :notify,
|
|
"test" => :stderr}
|
|
|
|
env = Rails.env
|
|
|
|
if defaults.key?(env)
|
|
msg = "You did not specify how you would like Rails to report " \
|
|
"deprecation notices for your #{env} environment, please " \
|
|
"set it to :#{defaults[env]} at config/environments/#{env}.rb"
|
|
|
|
warn msg
|
|
ActiveSupport::Deprecation.behavior = defaults[env]
|
|
else
|
|
msg = "You did not specify how you would like Rails to report " \
|
|
"deprecation notices for your #{env} environment, please " \
|
|
"set it to :log, :notify or :stderr at config/environments/#{env}.rb"
|
|
|
|
warn msg
|
|
ActiveSupport::Deprecation.behavior = :stderr
|
|
end
|
|
end
|
|
end
|
|
|
|
# Sets the default value for Time.zone
|
|
# If assigned value cannot be matched to a TimeZone, an exception will be raised.
|
|
initializer "active_support.initialize_time_zone" do |app|
|
|
require 'active_support/core_ext/time/zones'
|
|
zone_default = Time.__send__(:get_zone, app.config.time_zone)
|
|
|
|
unless zone_default
|
|
raise \
|
|
'Value assigned to config.time_zone not recognized.' +
|
|
'Run "rake -D time" for a list of tasks for finding appropriate time zone names.'
|
|
end
|
|
|
|
Time.zone_default = zone_default
|
|
end
|
|
end
|
|
end |