mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
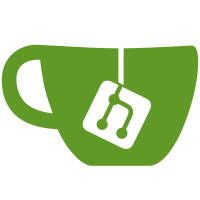
This PR ensures that when you're generating a scaffold or model and that model should belong to another database it will create an abstract class if it doesn't already exist. The new abstract class will ensure that the new model inherits from that class, but will not be deleted if the scaffold is deleted. This is because Rails can't know if you have other models inheriting from that class so we don't want to revoke that if the scaffold is destroyed. If the abstract class already exists it won't be created twice. If the options for `parent` are set, the generator will use that as the abstract class instead of creating one. The generated abstract class will add the writing connection automatically, users need to add the reading connection themselves as Rails doesn't know which is the reading connection. Co-authored-by: John Crepezzi <john.crepezzi@gmail.com>
35 lines
961 B
Ruby
35 lines
961 B
Ruby
# frozen_string_literal: true
|
|
|
|
require "isolation/abstract_unit"
|
|
require "env_helpers"
|
|
|
|
module ApplicationTests
|
|
class MultiDbRakeTest < ActiveSupport::TestCase
|
|
include ActiveSupport::Testing::Isolation, EnvHelpers
|
|
|
|
def setup
|
|
build_app(multi_db: true)
|
|
@output = rails("generate", "scaffold", "Pet", "name:string", "--database=animals")
|
|
end
|
|
|
|
def teardown
|
|
teardown_app
|
|
end
|
|
|
|
def test_generate_scaffold_creates_abstract_model
|
|
assert_match %r{app/models/pet\.rb}, @output
|
|
assert_match %r{app/models/animals_record\.rb}, @output
|
|
end
|
|
|
|
def test_destroy_scaffold_doesnt_remove_abstract_model
|
|
output = rails("destroy", "scaffold", "Pet", "--database=animals")
|
|
|
|
assert_match %r{app/models/pet\.rb}, output
|
|
assert_no_match %r{app/models/animals_record\.rb}, output
|
|
end
|
|
|
|
def test_creates_a_directory_for_migrations
|
|
assert_match %r{db/animals_migrate/}, @output
|
|
end
|
|
end
|
|
end
|