mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
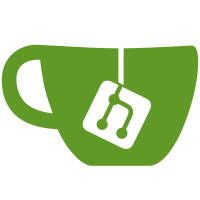
- This is similar to other railties blocks (such as `console`, `tasks` ...). The goal of this block is to allow the application or a railtie to load code after the server start. The use case can be to fire the webpack or react server in development or start some job worker like sidekiq or resque. Right now, all these tasks needs to be done in a separate shell and gem maintainer needs to add documentation on how to run their libraries if another program needs to run next to the Rails server. This feature can be used like this: ```ruby class SuperRailtie < Rails::Railtie server do WebpackServer.run end end ```
78 lines
2 KiB
Ruby
78 lines
2 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "isolation/abstract_unit"
|
|
require "console_helpers"
|
|
require "rails/command"
|
|
require "rails/commands/server/server_command"
|
|
|
|
module ApplicationTests
|
|
class ServerTest < ActiveSupport::TestCase
|
|
include ActiveSupport::Testing::Isolation
|
|
include ConsoleHelpers
|
|
|
|
def setup
|
|
build_app
|
|
end
|
|
|
|
def teardown
|
|
teardown_app
|
|
end
|
|
|
|
test "restart rails server with custom pid file path" do
|
|
skip "PTY unavailable" unless available_pty?
|
|
|
|
File.open("#{app_path}/config/boot.rb", "w") do |f|
|
|
f.puts "ENV['BUNDLE_GEMFILE'] = '#{Bundler.default_gemfile}'"
|
|
f.puts 'require "bundler/setup"'
|
|
end
|
|
|
|
primary, replica = PTY.open
|
|
pid = nil
|
|
|
|
Bundler.with_original_env do
|
|
pid = Process.spawn("bin/rails server -b localhost -P tmp/dummy.pid", chdir: app_path, in: replica, out: replica, err: replica)
|
|
assert_output("Listening", primary)
|
|
|
|
rails("restart")
|
|
|
|
assert_output("Restarting", primary)
|
|
assert_output("Listening", primary)
|
|
ensure
|
|
kill(pid) if pid
|
|
end
|
|
end
|
|
|
|
test "run +server+ blocks after the server starts" do
|
|
skip "PTY unavailable" unless available_pty?
|
|
|
|
File.open("#{app_path}/config/boot.rb", "w") do |f|
|
|
f.puts "ENV['BUNDLE_GEMFILE'] = '#{Bundler.default_gemfile}'"
|
|
f.puts 'require "bundler/setup"'
|
|
end
|
|
|
|
add_to_config(<<~CODE)
|
|
server do
|
|
puts 'Hello world'
|
|
end
|
|
CODE
|
|
|
|
primary, replica = PTY.open
|
|
pid = nil
|
|
|
|
Bundler.with_original_env do
|
|
pid = Process.spawn("bin/rails server -b localhost", chdir: app_path, in: replica, out: primary, err: replica)
|
|
assert_output("Hello world", primary)
|
|
assert_output("Listening", primary)
|
|
ensure
|
|
kill(pid) if pid
|
|
end
|
|
end
|
|
|
|
private
|
|
def kill(pid)
|
|
Process.kill("TERM", pid)
|
|
Process.wait(pid)
|
|
rescue Errno::ESRCH
|
|
end
|
|
end
|
|
end
|