mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
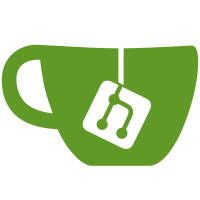
The priority wasn't being passed from ActiveJob to Backburner, despite priority being supported. This also brings it inline with the docs, which mark Backburner as supporting priorities in the "Backend Features" table: https://api.rubyonrails.org/classes/ActiveJob/QueueAdapters.html
36 lines
1.1 KiB
Ruby
36 lines
1.1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "backburner"
|
|
|
|
module ActiveJob
|
|
module QueueAdapters
|
|
# == Backburner adapter for Active Job
|
|
#
|
|
# Backburner is a beanstalkd-powered job queue that can handle a very
|
|
# high volume of jobs. You create background jobs and place them on
|
|
# multiple work queues to be processed later. Read more about
|
|
# Backburner {here}[https://github.com/nesquena/backburner].
|
|
#
|
|
# To use Backburner set the queue_adapter config to +:backburner+.
|
|
#
|
|
# Rails.application.config.active_job.queue_adapter = :backburner
|
|
class BackburnerAdapter
|
|
def enqueue(job) #:nodoc:
|
|
Backburner::Worker.enqueue(JobWrapper, [job.serialize], queue: job.queue_name, pri: job.priority)
|
|
end
|
|
|
|
def enqueue_at(job, timestamp) #:nodoc:
|
|
delay = timestamp - Time.current.to_f
|
|
Backburner::Worker.enqueue(JobWrapper, [job.serialize], queue: job.queue_name, pri: job.priority, delay: delay)
|
|
end
|
|
|
|
class JobWrapper #:nodoc:
|
|
class << self
|
|
def perform(job_data)
|
|
Base.execute job_data
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|