mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
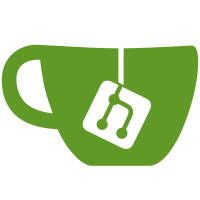
Fix: #36065 The IamgeAnalyzer passes a image to ImageMagick without checking if the image is supported by ImageMagick. This patch checks that image is supported and if not logs an error and returns an empty hash instead of raising an error. This is the same error handling we do when we encounter a LoadError when mini_magick is not installed.
40 lines
1.2 KiB
Ruby
40 lines
1.2 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "test_helper"
|
|
require "database/setup"
|
|
|
|
require "active_storage/analyzer/image_analyzer"
|
|
|
|
class ActiveStorage::Analyzer::ImageAnalyzerTest < ActiveSupport::TestCase
|
|
test "analyzing a JPEG image" do
|
|
blob = create_file_blob(filename: "racecar.jpg", content_type: "image/jpeg")
|
|
metadata = extract_metadata_from(blob)
|
|
|
|
assert_equal 4104, metadata[:width]
|
|
assert_equal 2736, metadata[:height]
|
|
end
|
|
|
|
test "analyzing a rotated JPEG image" do
|
|
blob = create_file_blob(filename: "racecar_rotated.jpg", content_type: "image/jpeg")
|
|
metadata = extract_metadata_from(blob)
|
|
|
|
assert_equal 2736, metadata[:width]
|
|
assert_equal 4104, metadata[:height]
|
|
end
|
|
|
|
test "analyzing an SVG image without an XML declaration" do
|
|
blob = create_file_blob(filename: "icon.svg", content_type: "image/svg+xml")
|
|
metadata = extract_metadata_from(blob)
|
|
|
|
assert_equal 792, metadata[:width]
|
|
assert_equal 584, metadata[:height]
|
|
end
|
|
|
|
test "analyzing an unsupported image type" do
|
|
blob = create_blob(data: "bad", filename: "bad_file.bad", content_type: "image/bad_type")
|
|
metadata = extract_metadata_from(blob)
|
|
|
|
assert_nil metadata[:width]
|
|
assert_nil metadata[:heigh]
|
|
end
|
|
end
|