mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
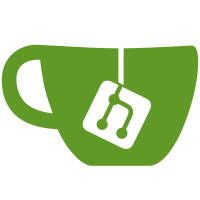
Previously we just stored handler, format, and variant and assigned a default format if none existed. Now we want to also store locale, and move the default format behaviour into unbound template.
44 lines
1,000 B
Ruby
44 lines
1,000 B
Ruby
# frozen_string_literal: true
|
|
|
|
require "concurrent/map"
|
|
|
|
module ActionView
|
|
class UnboundTemplate
|
|
attr_reader :handler, :format, :variant, :locale, :virtual_path
|
|
|
|
def initialize(source, identifier, handler, format:, variant:, locale:, virtual_path:)
|
|
@source = source
|
|
@identifier = identifier
|
|
@handler = handler
|
|
|
|
@format = format
|
|
@variant = variant
|
|
@locale = locale
|
|
@virtual_path = virtual_path
|
|
|
|
@templates = Concurrent::Map.new(initial_capacity: 2)
|
|
end
|
|
|
|
def bind_locals(locals)
|
|
@templates[locals] ||= build_template(locals)
|
|
end
|
|
|
|
private
|
|
def build_template(locals)
|
|
handler = Template.handler_for_extension(@handler)
|
|
format = @format || handler.try(:default_format)
|
|
|
|
Template.new(
|
|
@source,
|
|
@identifier,
|
|
handler,
|
|
|
|
format: format,
|
|
variant: @variant,
|
|
virtual_path: @virtual_path,
|
|
|
|
locals: locals
|
|
)
|
|
end
|
|
end
|
|
end
|