mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
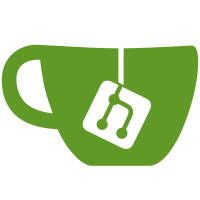
The current code base is not uniform. After some discussion, we have chosen to go with double quotes by default.
54 lines
1.1 KiB
Ruby
54 lines
1.1 KiB
Ruby
require "abstract_unit"
|
|
require "active_support/subscriber"
|
|
|
|
class TestSubscriber < ActiveSupport::Subscriber
|
|
attach_to :doodle
|
|
|
|
cattr_reader :events
|
|
|
|
def self.clear
|
|
@@events = []
|
|
end
|
|
|
|
def open_party(event)
|
|
events << event
|
|
end
|
|
|
|
private
|
|
|
|
def private_party(event)
|
|
events << event
|
|
end
|
|
end
|
|
|
|
# Monkey patch subscriber to test that only one subscriber per method is added.
|
|
class TestSubscriber
|
|
remove_method :open_party
|
|
def open_party(event)
|
|
events << event
|
|
end
|
|
end
|
|
|
|
class SubscriberTest < ActiveSupport::TestCase
|
|
def setup
|
|
TestSubscriber.clear
|
|
end
|
|
|
|
def test_attaches_subscribers
|
|
ActiveSupport::Notifications.instrument("open_party.doodle")
|
|
|
|
assert_equal "open_party.doodle", TestSubscriber.events.first.name
|
|
end
|
|
|
|
def test_attaches_only_one_subscriber
|
|
ActiveSupport::Notifications.instrument("open_party.doodle")
|
|
|
|
assert_equal 1, TestSubscriber.events.size
|
|
end
|
|
|
|
def test_does_not_attach_private_methods
|
|
ActiveSupport::Notifications.instrument("private_party.doodle")
|
|
|
|
assert_equal [], TestSubscriber.events
|
|
end
|
|
end
|