mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
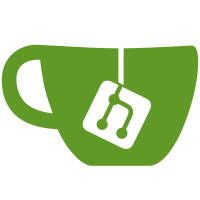
In https://github.com/rails/rails/pull/28676 the `#to_path` method was added to `ActionDispatch::Http::UploadedFile`. This broke usage with `IO.copy_stream`: source = ActionDispatch::Http::UploadedFile.new(...) IO.copy_stream(source, destination) # ~> TypeError: can't convert ActionDispatch::Http::UploadedFile to IO (ActionDispatch::Http::UploadedFile#to_io gives Tempfile) Normally `IO.copy_stream` just calls `#read` on the source object. However, when `#to_path` is defined, `IO.copy_stream` calls `#to_io` in order to retrieve the raw `File` object. In that case it trips up, because `ActionDispatch::Http::UploadedFile#to_io` returned a `Tempfile` object, which is not an `IO` subclass. We fix this by having `#to_io` return an actual `File` object.
92 lines
2.3 KiB
Ruby
92 lines
2.3 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
module ActionDispatch
|
|
module Http
|
|
# Models uploaded files.
|
|
#
|
|
# The actual file is accessible via the +tempfile+ accessor, though some
|
|
# of its interface is available directly for convenience.
|
|
#
|
|
# Uploaded files are temporary files whose lifespan is one request. When
|
|
# the object is finalized Ruby unlinks the file, so there is no need to
|
|
# clean them with a separate maintenance task.
|
|
class UploadedFile
|
|
# The basename of the file in the client.
|
|
attr_accessor :original_filename
|
|
|
|
# A string with the MIME type of the file.
|
|
attr_accessor :content_type
|
|
|
|
# A +Tempfile+ object with the actual uploaded file. Note that some of
|
|
# its interface is available directly.
|
|
attr_accessor :tempfile
|
|
|
|
# A string with the headers of the multipart request.
|
|
attr_accessor :headers
|
|
|
|
def initialize(hash) # :nodoc:
|
|
@tempfile = hash[:tempfile]
|
|
raise(ArgumentError, ":tempfile is required") unless @tempfile
|
|
|
|
if hash[:filename]
|
|
@original_filename = hash[:filename].dup
|
|
|
|
begin
|
|
@original_filename.encode!(Encoding::UTF_8)
|
|
rescue EncodingError
|
|
@original_filename.force_encoding(Encoding::UTF_8)
|
|
end
|
|
else
|
|
@original_filename = nil
|
|
end
|
|
|
|
@content_type = hash[:type]
|
|
@headers = hash[:head]
|
|
end
|
|
|
|
# Shortcut for +tempfile.read+.
|
|
def read(length = nil, buffer = nil)
|
|
@tempfile.read(length, buffer)
|
|
end
|
|
|
|
# Shortcut for +tempfile.open+.
|
|
def open
|
|
@tempfile.open
|
|
end
|
|
|
|
# Shortcut for +tempfile.close+.
|
|
def close(unlink_now = false)
|
|
@tempfile.close(unlink_now)
|
|
end
|
|
|
|
# Shortcut for +tempfile.path+.
|
|
def path
|
|
@tempfile.path
|
|
end
|
|
|
|
# Shortcut for +tempfile.to_path+.
|
|
def to_path
|
|
@tempfile.to_path
|
|
end
|
|
|
|
# Shortcut for +tempfile.rewind+.
|
|
def rewind
|
|
@tempfile.rewind
|
|
end
|
|
|
|
# Shortcut for +tempfile.size+.
|
|
def size
|
|
@tempfile.size
|
|
end
|
|
|
|
# Shortcut for +tempfile.eof?+.
|
|
def eof?
|
|
@tempfile.eof?
|
|
end
|
|
|
|
def to_io
|
|
@tempfile.to_io
|
|
end
|
|
end
|
|
end
|
|
end
|