mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
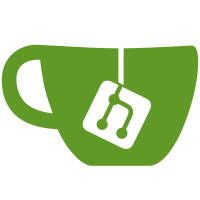
This implements several changes to encourage deterministic encryption to remain unchanged. The main motivation is letting you define unique indexes on deterministically-encrypted columns: - By default, deterministic encryption will always use the oldest encryption scheme to encrypt new data, when there are many. - You can skip this default behavior and make it always use the current encryption scheme with: ```ruby deterministic: { fixed: false } # using this should be a rare need ``` - Deterministic encryption still supports previous encryption schemes normally. So they will be used to add additional values to queries, for example. - You can't rotate deterministic encryption keys anymore. We can add support for that in the future. This makes for reasonable defaults: - People using "deterministic: true" will get unique indexes working out of the box. - The system will encourage keeping deterministic encryption stable: - By always using oldest encryption schemes - By forbidding configuring multiple keys But you can still opt-out of the default if you need to.
66 lines
1.9 KiB
Ruby
66 lines
1.9 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "cases/encryption/helper"
|
|
|
|
class ActiveRecord::EncryptionPropertiesTest < ActiveRecord::EncryptionTestCase
|
|
setup do
|
|
@properties = ActiveRecord::Encryption::Properties.new
|
|
end
|
|
|
|
test "behaves like a hash" do
|
|
@properties[:key_1] = "value 1"
|
|
@properties[:key_2] = "value 2"
|
|
|
|
assert_equal "value 1", @properties[:key_1]
|
|
assert_equal "value 2", @properties[:key_2]
|
|
end
|
|
|
|
test "defines custom accessors for some default properties" do
|
|
auth_tag = "some auth tag"
|
|
|
|
@properties.auth_tag = auth_tag
|
|
assert_equal auth_tag, @properties.auth_tag
|
|
assert_equal auth_tag, @properties[:at]
|
|
end
|
|
|
|
test "raises EncryptedContentIntegrity when trying to override properties" do
|
|
@properties[:key_1] = "value 1"
|
|
|
|
assert_raises ActiveRecord::Encryption::Errors::EncryptedContentIntegrity do
|
|
@properties[:key_1] = "value 1"
|
|
end
|
|
end
|
|
|
|
test "add will add all the properties passed" do
|
|
@properties.add(key_1: "value 1", key_2: "value 2")
|
|
|
|
assert_equal "value 1", @properties[:key_1]
|
|
assert_equal "value 2", @properties[:key_2]
|
|
end
|
|
|
|
test "validate allowed types on creation" do
|
|
example_of_valid_values.each do |value|
|
|
ActiveRecord::Encryption::Properties.new(some_value: value)
|
|
end
|
|
|
|
assert_raises ActiveRecord::Encryption::Errors::ForbiddenClass do
|
|
ActiveRecord::Encryption::Properties.new(my_class: MyClass.new)
|
|
end
|
|
end
|
|
|
|
test "validate allowed_types setting headers" do
|
|
example_of_valid_values.each.with_index do |value, index|
|
|
@properties["some_value_#{index}"] = value
|
|
end
|
|
|
|
assert_raises ActiveRecord::Encryption::Errors::ForbiddenClass do
|
|
@properties["some_value"] = MyClass.new
|
|
end
|
|
end
|
|
|
|
MyClass = Struct.new(:some_value)
|
|
|
|
def example_of_valid_values
|
|
["a string", 123, 123.5, true, false, nil, :a_symbol, ActiveRecord::Encryption::Message.new]
|
|
end
|
|
end
|