mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
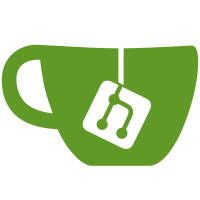
When dealing with the "details" for a template: locale, format, variant, and handler, previously we would store these in an ad-hoc way every place we did. Often as a hash or as separate instance variables on a class. This PR attempts to simplify this by encapsulating known details on a template in a new ActionView::TemplateDetails class, and requested details in ActionView::TemplateDetails::Requested. This allowed extracting and simplifying filtering and sorting logic from the Resolver class as well as extracting default format logic from UnboundTemplate. As well as reducing complexity, in the future this should make it possible to provide suggestions on missing template errors due to mismatched details, and might allow improved performance. At least for now these new classes are private (:nodoc) Co-authored-by: John Crepezzi <john.crepezzi@gmail.com>
38 lines
859 B
Ruby
38 lines
859 B
Ruby
# frozen_string_literal: true
|
|
|
|
require "concurrent/map"
|
|
|
|
module ActionView
|
|
class UnboundTemplate
|
|
attr_reader :virtual_path, :details
|
|
delegate :locale, :format, :variant, :handler, to: :@details
|
|
|
|
def initialize(source, identifier, details:, virtual_path:)
|
|
@source = source
|
|
@identifier = identifier
|
|
@details = details
|
|
@virtual_path = virtual_path
|
|
|
|
@templates = Concurrent::Map.new(initial_capacity: 2)
|
|
end
|
|
|
|
def bind_locals(locals)
|
|
@templates[locals] ||= build_template(locals)
|
|
end
|
|
|
|
private
|
|
def build_template(locals)
|
|
Template.new(
|
|
@source,
|
|
@identifier,
|
|
details.handler_class,
|
|
|
|
format: details.format_or_default,
|
|
variant: variant&.to_s,
|
|
virtual_path: @virtual_path,
|
|
|
|
locals: locals
|
|
)
|
|
end
|
|
end
|
|
end
|