mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
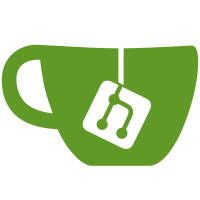
Before: ``` Topic Update All (0.4ms) UPDATE `topics` SET `topics`.`replies_count` = COALESCE(`topics`.`replies_count`, 0) + 1, `topics`.`updated_at` = '2018-09-27 18:34:05.068774' WHERE `topics`.`id` = ? [["id", 7]] ``` After: ``` Topic Update All (0.4ms) UPDATE `topics` SET `topics`.`replies_count` = COALESCE(`topics`.`replies_count`, 0) + ?, `topics`.`updated_at` = ? WHERE `topics`.`id` = ? [["replies_count", 1], ["updated_at", 2018-09-27 18:55:05 UTC], ["id", 7]] ```
49 lines
951 B
Ruby
49 lines
951 B
Ruby
# frozen_string_literal: true
|
|
|
|
module Arel # :nodoc: all
|
|
###
|
|
# Methods for creating various nodes
|
|
module FactoryMethods
|
|
def create_true
|
|
Arel::Nodes::True.new
|
|
end
|
|
|
|
def create_false
|
|
Arel::Nodes::False.new
|
|
end
|
|
|
|
def create_table_alias(relation, name)
|
|
Nodes::TableAlias.new(relation, name)
|
|
end
|
|
|
|
def create_join(to, constraint = nil, klass = Nodes::InnerJoin)
|
|
klass.new(to, constraint)
|
|
end
|
|
|
|
def create_string_join(to)
|
|
create_join to, nil, Nodes::StringJoin
|
|
end
|
|
|
|
def create_and(clauses)
|
|
Nodes::And.new clauses
|
|
end
|
|
|
|
def create_on(expr)
|
|
Nodes::On.new expr
|
|
end
|
|
|
|
def grouping(expr)
|
|
Nodes::Grouping.new expr
|
|
end
|
|
|
|
###
|
|
# Create a LOWER() function
|
|
def lower(column)
|
|
Nodes::NamedFunction.new "LOWER", [Nodes.build_quoted(column)]
|
|
end
|
|
|
|
def coalesce(*exprs)
|
|
Nodes::NamedFunction.new "COALESCE", exprs
|
|
end
|
|
end
|
|
end
|