mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
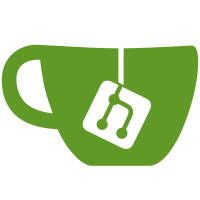
The implementation of the `CSV` shortcut method is broken in Ruby 3 for calls that look like this: ```ruby CSV(write_stream, col_sep: "|", headers: headers, write_headers: true) do |csv| ... end ``` The above will result in the following error when the `CSV` method attempts to pass on arguments to `CSV#instance`: ``` ArgumentError: wrong number of arguments (given 2, expected 0..1) ``` The issue is due to the changes in Ruby 3 relating to positional & keyword arguments. This commit updates the `CSV()` shortcut implementation to work with Ruby 3, and also updates the documentation for the shortcut method. https://github.com/ruby/csv/commit/310dee45fa
124 lines
2.4 KiB
Ruby
124 lines
2.4 KiB
Ruby
# frozen_string_literal: false
|
|
|
|
require_relative "../helper"
|
|
|
|
class TestCSVInterfaceReadWrite < Test::Unit::TestCase
|
|
extend DifferentOFS
|
|
|
|
def test_filter
|
|
input = <<-CSV.freeze
|
|
1;2;3
|
|
4;5
|
|
CSV
|
|
output = ""
|
|
CSV.filter(input, output,
|
|
in_col_sep: ";",
|
|
out_col_sep: ",",
|
|
converters: :all) do |row|
|
|
row.map! {|n| n * 2}
|
|
row << "Added\r"
|
|
end
|
|
assert_equal(<<-CSV, output)
|
|
2,4,6,"Added\r"
|
|
8,10,"Added\r"
|
|
CSV
|
|
end
|
|
|
|
def test_filter_headers_true
|
|
input = <<-CSV.freeze
|
|
Name,Value
|
|
foo,0
|
|
bar,1
|
|
baz,2
|
|
CSV
|
|
output = ""
|
|
CSV.filter(input, output, headers: true) do |row|
|
|
row[0] += "X"
|
|
row[1] = row[1].to_i + 1
|
|
end
|
|
assert_equal(<<-CSV, output)
|
|
fooX,1
|
|
barX,2
|
|
bazX,3
|
|
CSV
|
|
end
|
|
|
|
def test_filter_headers_true_write_headers
|
|
input = <<-CSV.freeze
|
|
Name,Value
|
|
foo,0
|
|
bar,1
|
|
baz,2
|
|
CSV
|
|
output = ""
|
|
CSV.filter(input, output, headers: true, out_write_headers: true) do |row|
|
|
if row.is_a?(Array)
|
|
row[0] += "X"
|
|
row[1] += "Y"
|
|
else
|
|
row[0] += "X"
|
|
row[1] = row[1].to_i + 1
|
|
end
|
|
end
|
|
assert_equal(<<-CSV, output)
|
|
NameX,ValueY
|
|
fooX,1
|
|
barX,2
|
|
bazX,3
|
|
CSV
|
|
end
|
|
|
|
def test_filter_headers_array_write_headers
|
|
input = <<-CSV.freeze
|
|
foo,0
|
|
bar,1
|
|
baz,2
|
|
CSV
|
|
output = ""
|
|
CSV.filter(input, output,
|
|
headers: ["Name", "Value"],
|
|
out_write_headers: true) do |row|
|
|
row[0] += "X"
|
|
row[1] = row[1].to_i + 1
|
|
end
|
|
assert_equal(<<-CSV, output)
|
|
Name,Value
|
|
fooX,1
|
|
barX,2
|
|
bazX,3
|
|
CSV
|
|
end
|
|
|
|
def test_instance_same
|
|
data = ""
|
|
assert_equal(CSV.instance(data, col_sep: ";").object_id,
|
|
CSV.instance(data, col_sep: ";").object_id)
|
|
end
|
|
|
|
def test_instance_append
|
|
output = ""
|
|
CSV.instance(output, col_sep: ";") << ["a", "b", "c"]
|
|
assert_equal(<<-CSV, output)
|
|
a;b;c
|
|
CSV
|
|
CSV.instance(output, col_sep: ";") << [1, 2, 3]
|
|
assert_equal(<<-CSV, output)
|
|
a;b;c
|
|
1;2;3
|
|
CSV
|
|
end
|
|
|
|
def test_instance_shortcut
|
|
assert_equal(CSV.instance,
|
|
CSV {|csv| csv})
|
|
end
|
|
|
|
def test_instance_shortcut_with_io
|
|
io = StringIO.new
|
|
from_instance = CSV.instance(io, col_sep: ";") { |csv| csv << ["a", "b", "c"] }
|
|
from_shortcut = CSV(io, col_sep: ";") { |csv| csv << ["e", "f", "g"] }
|
|
|
|
assert_equal(from_instance, from_shortcut)
|
|
assert_equal(from_instance.string, "a;b;c\ne;f;g\n")
|
|
end
|
|
end
|